js如何将数组写入Json文件
时间: 2024-12-21 09:29:11 浏览: 5
在JavaScript中,要将数组写入JSON文件,你可以使用`fs`模块(Node.js环境)或浏览器的`fetch` API(配合Blob和File API)。以下是两种方式:
1. 使用Node.js的`fs`模块[^1]:
```javascript
const fs = require('fs');
let arr = [/* your array */];
try {
const jsonStr = JSON.stringify(arr);
fs.writeFile('array.json', jsonStr, (err) => {
if (err) throw err;
console.log('Array written to file successfully.');
});
} catch (error) {
console.error('Error writing to file:', error);
}
```
2. 使用浏览器的`fetch` API:
```javascript
async function writeJsonToFile(array) {
try {
const data = JSON.stringify(array);
const blob = new Blob([data], { type: "application/json" });
const url = URL.createObjectURL(blob);
await fetch('your-server-url-or-local-file-path', {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: blob
});
URL.revokeObjectURL(url);
console.log('Array written to server successfully.');
} catch (error) {
console.error('Error writing to file:', error);
}
}
// Call the function with your array
writeJsonToFile(yourArray);
```
在这个例子中,`fetch`用于向服务器端发送PUT请求,将JSON数据作为文件内容。
阅读全文
相关推荐
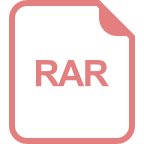
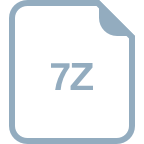
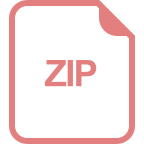



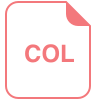

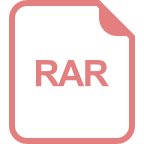
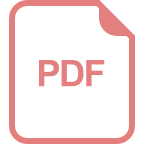
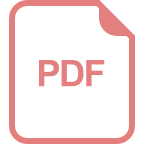
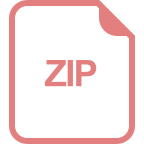
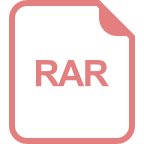
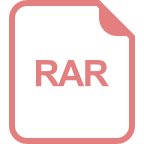
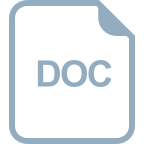
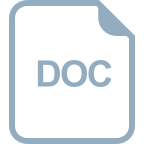
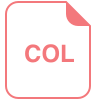


