根据科罗拉多州罗斯国家森林区域树木类型的观测数据 covtype.csv.,用决策树算法实现树木类型识别任务(python代码),实验结果需要量化与可视化结果 注意: 后58000条数据为测试集
时间: 2024-12-27 11:31:43 浏览: 3
首先,为了实现这个任务,我们需要做以下几个步骤:
1. **加载数据**:使用pandas库读取`covtype.csv`文件,并了解数据结构。
```python
import pandas as pd
# 加载数据
url = "https://archive.ics.uci.edu/ml/machine-learning-databases/covtype/covtype.data"
column_names = ['elevation', 'aspect', 'slope', 'horizontal_distance_to_hydrology', 'vertical_distance_to_hydrology', 'horizontal_distance_to_roadways', 'hillshade_9am', 'hillshade_noon', 'hillshade_3pm', 'horizontal_diameter_at Breast_height', 'deciduous_forest', 'coniferous_forest', 'mixed_forest', 'open_pine_forest', 'closed_pine_forest', 'lodgepole_pine forest', 'aspen', 'Douglas_fir', 'kneedle_pine', 'Ponderosa_pine', 'cottonwood_willow', 'willow', 'juniper_pinyon', 'mesquite', 'birch', 'maple', 'oak', 'pine', 'spruce', 'grass_pasture', 'sod_farm', 'urban_and_residential', 'barren_land', 'lake', 'pond', 'stream', 'ravine', 'Other']
data = pd.read_csv(url, header=None, names=column_names)
```
2. **预处理数据**:将类别特征转换为数值,划分训练集和测试集。
```python
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
# 对分类变量进行编码
le = LabelEncoder()
for col in data.columns[14:]:
data[col] = le.fit_transform(data[col])
# 划分训练集和测试集
X = data.iloc[:, :-1]
y = data['Cover_Type']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.5, random_state=42, shuffle=True, stratify=y)
```
3. **构建决策树模型**:
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import classification_report, confusion_matrix, accuracy_score
# 创建并训练决策树模型
model = DecisionTreeClassifier(random_state=42)
model.fit(X_train, y_train)
```
4. **评估与预测**:
```python
# 预测测试集
y_pred = model.predict(X_test)
# 量化结果
print("Accuracy:", accuracy_score(y_test, y_pred))
print("\nClassification Report:\n", classification_report(y_test, y_pred))
conf_mat = confusion_matrix(y_test, y_pred)
print("\nConfusion Matrix:\n", conf_mat)
```
5. **可视化结果**:
```python
import seaborn as sns
import matplotlib.pyplot as plt
# 可视化混淆矩阵
sns.heatmap(conf_mat, annot=True, cmap='Blues')
plt.xlabel('Predicted Classes')
plt.ylabel('True Classes');
plt.title('Decision Tree Confusion Matrix');
plt.show()
```
阅读全文
相关推荐
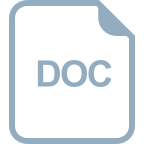
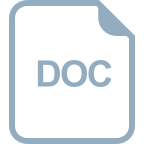
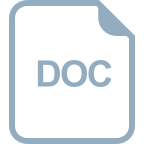



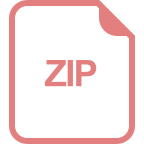
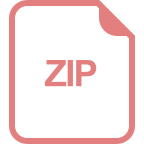
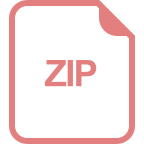
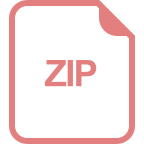
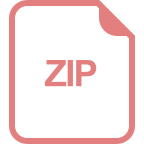
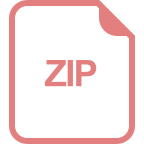
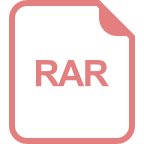
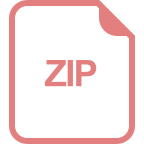
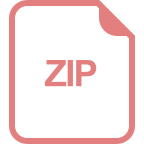
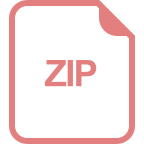
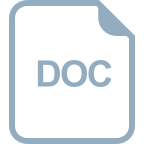