简要介绍迪杰斯特拉算法
时间: 2024-01-30 16:03:22 浏览: 205
迪杰斯特拉算法是一种用于解决有权图中最短路径问题的算法。它从一个起始点开始,通过贪心算法的策略,逐步扩展到其他顶点,直到找到到达目标顶点的最短路径。
算法的主要步骤如下:
1. 创建一个距离数组,用于存储从起始点到每个顶点的最短距离。初始时,起始点的距离为0,其他顶点的距离为无穷大。
2. 创建一个集合,用于存储已经找到最短路径的顶点。
3. 重复以下步骤,直到集合中包含所有顶点:
- 从距离数组中选择一个距离最小且不在集合中的顶点,将其加入集合。
- 更新距离数组中其他顶点的距离,如果通过当前顶点可以获得更短的路径。
4. 最终,距离数组中存储的就是从起始点到每个顶点的最短距离。
这是一个简要介绍迪杰斯特拉算法的过程。通过这个算法,我们可以找到从起始点到目标顶点的最短路径。
相关问题
实现迪杰斯特拉算法
好的,迪杰斯特拉算法是一种单源最短路径算法,其基本思想是从起点开始,逐步扩展最短路径,直到到达终点为止。下面是一个简单的实现示例(假设点的编号从0开始):
```python
import heapq
def dijkstra(graph, start):
# 初始化距离为无穷大
distances = [float('inf')] * len(graph)
# 起点的距离为0
distances[start] = 0
# 用堆来维护未处理的点
heap = [(0, start)]
while heap:
# 取出距离最小的点
(dist, node) = heapq.heappop(heap)
# 如果该点已经处理过,直接跳过
if dist > distances[node]:
continue
# 遍历所有的邻居,更新距离
for neighbor, weight in graph[node].items():
new_distance = dist + weight
# 如果新的距离更短,更新距离
if new_distance < distances[neighbor]:
distances[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
return distances
```
这个实现中,我们使用了一个堆来维护未处理的点,并且使用了一个数组来保存每个点的最短距离。算法的时间复杂度为O(ElogV),其中E为边数,V为点数。
迪杰斯特拉算法c语言
以下是一个简单的 C 语言实现迪杰斯特拉算法的代码示例:
```c
#include<stdio.h>
#define INF 9999
#define MAX 10
void dijkstra(int G[MAX][MAX], int n, int startnode);
int main() {
int G[MAX][MAX], i, j, n, u;
printf("Enter the number of vertices:");
scanf("%d", &n);
printf("\nEnter the adjacency matrix:\n");
for(i = 0; i < n; i++)
for(j = 0; j < n; j++)
scanf("%d", &G[i][j]);
printf("\nEnter the starting node:");
scanf("%d", &u);
dijkstra(G, n, u);
return 0;
}
void dijkstra(int G[MAX][MAX], int n, int startnode) {
int cost[MAX][MAX], distance[MAX], pred[MAX];
int visited[MAX], count, mindistance, nextnode, i, j;
// Creating cost matrix
for(i = 0; i < n; i++)
for(j = 0; j < n; j++)
if(G[i][j] == 0)
cost[i][j] = INF;
else
cost[i][j] = G[i][j];
// Initializing visited[], distance[], and pred[]
for(i = 0; i < n; i++) {
distance[i] = cost[startnode][i];
pred[i] = startnode;
visited[i] = 0;
}
distance[startnode] = 0;
visited[startnode] = 1;
count = 1;
while(count < n - 1) {
mindistance = INF;
// Nextnode gives the node at minimum distance
for(i = 0; i < n; i++)
if(distance[i] < mindistance && !visited[i]) {
mindistance = distance[i];
nextnode = i;
}
// Checking if a better path exists through nextnode
visited[nextnode] = 1;
for(i = 0; i < n; i++)
if(!visited[i])
if(mindistance + cost[nextnode][i] < distance[i]) {
distance[i] = mindistance + cost[nextnode][i];
pred[i] = nextnode;
}
count++;
}
// Printing the distance and path of each node
for(i = 0; i < n; i++)
if(i != startnode) {
printf("\nDistance of node %d = %d", i, distance[i]);
printf("\nPath = %d", i);
j = i;
do {
j = pred[j];
printf("<-%d", j);
} while(j != startnode);
}
}
```
注意:此代码仅为示例,实际应用中需要根据具体情况进行适当修改。
阅读全文
相关推荐
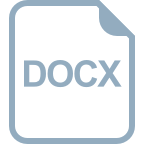
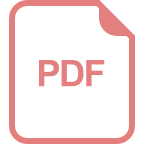
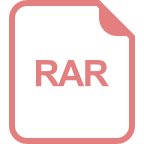








