aes-256-gcm js
时间: 2024-09-18 12:07:45 浏览: 70
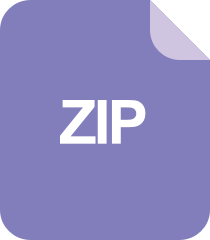
aes-256-gcm:静态类,可使用AES 256 GCM算法简化加密解密

AES-256-GCM是一种常用的对称加密算法,它结合了高级加密标准(Advanced Encryption Standard,AES)的256位密钥强度和 Galois/Counter Mode (GCM) 模式的安全功能。GCM提供了一种安全的模式来加密数据(包括消息体)以及生成与之关联的认证标签,用于验证数据完整性和未授权访问。
在JavaScript中,你可以通过各种库来利用AES-256-GCM。例如,`crypto-js`库就是一个流行的选项,它提供了易于使用的API来执行AES-256-GCM操作。以下是一个简单的示例:
```javascript
const CryptoJS = require('crypto-js');
// 加密函数
function encrypt(plaintext, key, iv) {
const encryptedData = CryptoJS.AES.encrypt(
plaintext,
key,
{ mode: CryptoJS.mode.GCM, iv: iv, padding: CryptoJS.pad.ZeroPadding, authenticationTagLength: 16 }
);
return encryptedData.toString();
}
// 解密函数
function decrypt(ciphertext, key, iv, authTag) {
try {
const decryptedData = CryptoJS.AES.decrypt(
ciphertext,
key,
{ mode: CryptoJS.mode.GCM, iv: iv, padding: CryptoJS.pad.ZeroPadding, authenticationTag: authTag }
);
return decryptedData.toString(CryptoJS.enc.Utf8);
} catch (e) {
// 处理错误
}
}
// 示例用法
const key = '256-bit-secret-key'; // 你的密钥,通常是十六进制字符串
const plaintext = 'Hello, world!';
const iv = CryptoJS.lib.WordArray.random(12); // 随机初始化向量
const ciphertext = encrypt(plaintext, key, iv);
const decryptedPlaintext = decrypt(ciphertext, key, iv);
console.log('Encrypted:', ciphertext);
console.log('Decrypted:', decryptedPlaintext);
```
阅读全文
相关推荐
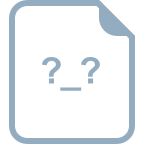
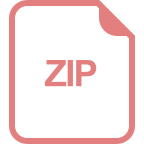

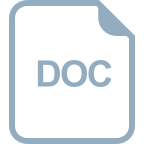

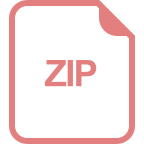
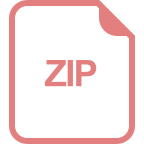


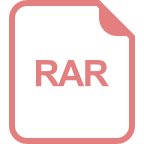
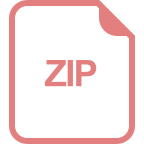
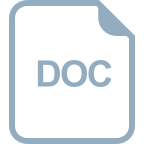
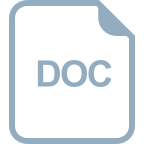
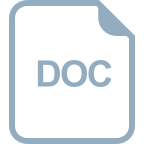
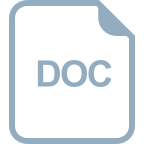

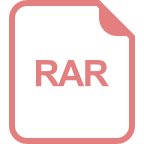
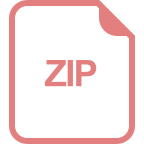