用Keras框架写出WOA优化LSTM参数进行多变量预测代码
时间: 2024-03-15 08:45:35 浏览: 185
以下是用Keras框架编写WOA优化LSTM参数进行多变量预测的代码:
首先,我们需要导入必要的库:
```python
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Dense, LSTM
from keras.optimizers import Adam
```
然后,我们需要定义一些超参数:
```python
n_features = 4 # 输入特征数
n_neurons = 50 # LSTM神经元数量
n_epochs = 100 # 训练轮数
batch_size = 32 # 批处理大小
```
接下来,我们需要定义WOA优化算法的相关函数:
```python
def woa_init_search_space(num_params, search_space):
return np.random.uniform(search_space[0], search_space[1], size=(num_params,))
def woa_get_fitness(model, X_train, y_train, X_valid, y_valid):
model.fit(X_train, y_train, epochs=n_epochs, batch_size=batch_size, verbose=0)
score = model.evaluate(X_valid, y_valid, verbose=0)
return score[0]
def woa_get_parameters(model):
return model.get_weights()
def woa_set_parameters(model, params):
model.set_weights(params)
def woa_search(model, X_train, y_train, X_valid, y_valid, search_space, max_iter=100):
lb, ub = search_space
num_params = len(model.get_weights())
best_fitness = float('inf')
best_params = None
a = 2
a2 = -1
for i in range(max_iter):
for j in range(num_params):
r1 = np.random.random()
r2 = np.random.random()
A = 2 * a * r1 - a
C = 2 * r2
p = np.random.random()
b = 1
l = (a2 - i) * (ub - lb) / max_iter + lb
if p < 0.5:
if abs(A) < 1:
D = abs(C * best_params[j] - model.get_weights()[j])
new_pos = best_params[j] - A * D
else:
rand_param = woa_init_search_space(1, [lb, ub])[0]
D = abs(C * rand_param - model.get_weights()[j])
new_pos = rand_param - A * D
else:
D = abs(best_params[j] - model.get_weights()[j])
new_pos = best_params[j] - b * D
if new_pos < lb:
new_pos = lb
elif new_pos > ub:
new_pos = ub
model.get_weights()[j] = new_pos
fitness = woa_get_fitness(model, X_train, y_train, X_valid, y_valid)
if fitness < best_fitness:
best_fitness = fitness
best_params = woa_get_parameters(model)
return best_params
```
现在,我们可以加载并准备数据:
```python
data = pd.read_csv('data.csv')
X = data.iloc[:, :n_features].values
y = data.iloc[:, n_features:].values
n_samples = len(data)
n_train = int(n_samples * 0.8)
X_train, y_train = X[:n_train], y[:n_train]
X_valid, y_valid = X[n_train:], y[n_train:]
```
然后,我们可以构建模型并使用WOA优化算法来优化参数:
```python
model = Sequential()
model.add(LSTM(n_neurons, input_shape=(None, n_features)))
model.add(Dense(y.shape[1]))
model.compile(optimizer=Adam(), loss='mse')
search_space = [-5, 5]
woa_params = woa_search(model, X_train.reshape(-1, 1, n_features), y_train, X_valid.reshape(-1, 1, n_features), y_valid, search_space)
woa_set_parameters(model, woa_params)
```
最后,我们可以使用训练好的模型来进行预测:
```python
X_test = np.array([[0.1, 0.2, 0.3, 0.4], [0.2, 0.3, 0.4, 0.5], [0.3, 0.4, 0.5, 0.6]])
y_pred = model.predict(X_test.reshape(-1, 1, n_features))
print(y_pred)
```
以上就是用Keras框架编写WOA优化LSTM参数进行多变量预测的完整代码。
阅读全文
相关推荐
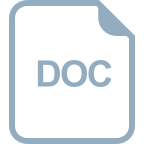
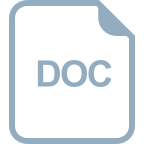
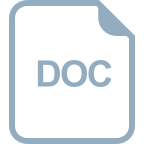

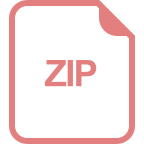
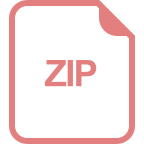
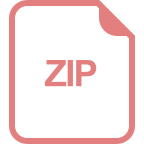
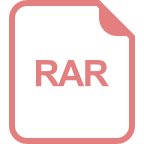
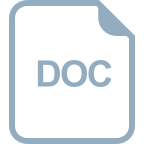
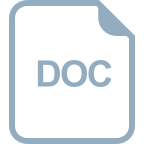








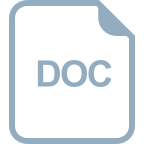
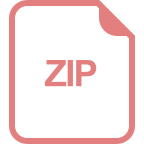