java文件夹上传保存文件夹记录到数据库如何保证不被重复创建文件夹数据,给出例子
时间: 2024-05-14 15:17:10 浏览: 87
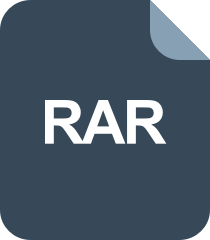
java保存文件到数据库

为避免重复创建文件夹数据,我们可以在数据库中为每个文件夹设置一个唯一的标识符。可以使用文件夹的名称或者路径来生成这个标识符。
在上传文件夹时,我们需要首先查询数据库中是否已经存在这个文件夹的记录。如果存在,就不需要创建新的记录了;如果不存在,我们就需要创建新的记录,并将文件夹的标识符保存到数据库中。
以下是一个示例代码,实现了文件夹上传时保存记录到数据库的功能(假设我们使用MySQL数据库):
```java
import java.sql.*;
import java.util.UUID;
public class FolderUploader {
public static void uploadFolder(String folderPath) {
// 首先查询数据库中是否已经存在这个文件夹的记录
String folderId = queryFolderId(folderPath);
if (folderId != null) {
System.out.println("Folder already exists in database: " + folderPath);
return;
}
// 如果不存在,就创建新的记录并保存到数据库中
folderId = UUID.randomUUID().toString(); // 使用UUID作为文件夹的唯一标识符
String folderName = folderPath.substring(folderPath.lastIndexOf('/') + 1);
String insertSql = "INSERT INTO folders (id, name, path) VALUES (?, ?, ?)";
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
PreparedStatement ps = conn.prepareStatement(insertSql)) {
ps.setString(1, folderId);
ps.setString(2, folderName);
ps.setString(3, folderPath);
ps.executeUpdate();
System.out.println("Folder uploaded successfully: " + folderPath);
} catch (SQLException e) {
e.printStackTrace();
System.out.println("Failed to upload folder: " + folderPath);
}
}
private static String queryFolderId(String folderPath) {
String selectSql = "SELECT id FROM folders WHERE path = ?";
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
PreparedStatement ps = conn.prepareStatement(selectSql)) {
ps.setString(1, folderPath);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
return rs.getString("id");
}
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
// 在这里调用 uploadFolder 方法上传文件夹
}
}
```
请注意,这只是一个示例代码,实际情况下还需要考虑更多的细节和异常情况。
阅读全文
相关推荐
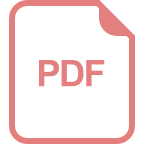
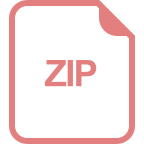
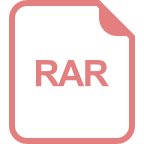
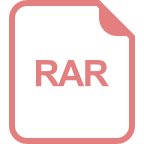
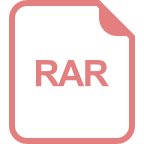
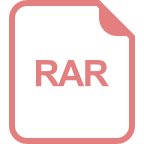
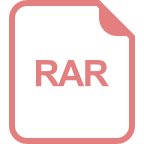
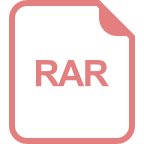
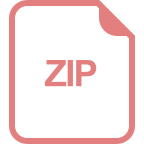
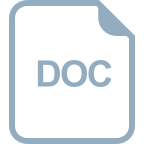
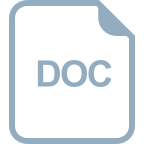
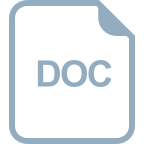
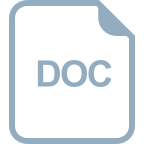
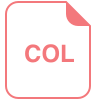

