分析这段代码给出详解 #define _GNU_SOURCE #include "sched.h" #include<sys/types.h> #include<sys/syscall.h> #include<unistd.h> #include <pthread.h> #include "stdio.h" #include "stdlib.h" #include "semaphore.h" #include "sys/wait.h" #include "string.h" int producer(void * args); int consumer(void * args); pthread_mutex_t mutex; sem_t product; sem_t warehouse; char buffer[8][4]; int bp=0; int main(int argc,char** argv){ pthread_mutex_init(&mutex,NULL);//初始化 sem_init(&product,0,0); sem_init(&warehouse,0,8); int clone_flag,arg,retval; char stack; //clone_flag=CLONE_SIGHAND|CLONE_VFORK //clone_flag=CLONE_VM|CLONE_FILES|CLONE_FS|CLONE_SIGHAND; clone_flag=CLONE_VM|CLONE_SIGHAND|CLONE_FS| CLONE_FILES; //printf("clone_flag=%d\n",clone_flag); int i; for(i=0;i<2;i++){ //创建四个线程 arg = i; //printf("arg=%d\n",(arg)); stack =(char*)malloc(4096); retval=clone(producer,&(stack[4095]),clone_flag,(void*)&arg); //printf("retval=%d\n",retval); stack=(char*)malloc(4096); retval=clone(consumer,&(stack[4095]),clone_flag,(void*)&arg); //printf("retval=%d\n\n",retval); usleep(1); } exit(1); } int producer(void *args){ int id = ((int)args); int i; for(i=0;i<10;i++){ sleep(i+1); //表现线程速度差别 sem_wait(&warehouse); pthread_mutex_lock(&mutex); if(id==0) strcpy(buffer[bp],"aaa/0"); else strcpy(buffer[bp],"bbb/0"); bp++; printf("producer %d produce %s in %d\n",id,buffer[bp-1],bp-1); pthread_mutex_unlock(&mutex); sem_post(&product); } printf("producer %d is over!\n",id); exit(id); } int consumer(void *args){ int id = ((int)args); int i; for(i=0;i<10;i++) { sleep(10-i); //表现线程速度差别 sem_wait(&product); pthread_mutex_lock(&mutex); bp--; printf("consumer %d get %s in %d\n",id,buffer[bp],bp+1); strcpy(buffer[bp],"zzz\0"); pthread_mutex_unlock(&mutex); sem_post(&warehouse); } printf("consumer %d is over!\n",id); exit(id); }
时间: 2024-03-04 11:50:46 浏览: 24
这段代码是一个生产者-消费者问题的解决方案,通过多线程实现。主要包括以下内容:
1. 引入了相关的头文件,包括sched.h、sys/types.h、sys/syscall.h、unistd.h、pthread.h、stdio.h、stdlib.h、semaphore.h和sys/wait.h等。
2. 定义了一些全局变量,包括一个互斥锁pthread_mutex_t mutex,两个信号量sem_t product和sem_t warehouse,以及一个二维字符数组buffer和一个变量bp,用于存储生产者生产的数据和消费者消费的数据。
3. 在main函数中初始化互斥锁和信号量,然后使用clone函数创建了两个生产者线程和两个消费者线程。
4. 生产者线程通过调用producer函数实现生产数据,每个线程生产10个数据,速度不同。生产者线程会先等待空闲的仓库,然后获取互斥锁,将生产的数据存入缓冲区中,再释放互斥锁,发送信号量通知消费者线程可以取数据了。
5. 消费者线程通过调用consumer函数实现消费数据,每个线程消费10个数据,速度不同。消费者线程会先等待有可用的数据,然后获取互斥锁,从缓冲区中取出数据,再将缓冲区中的数据清空,最后释放互斥锁,发送信号量通知生产者线程可以继续生产数据了。
6. 程序运行结束后,主线程退出。
需要注意的是,这段代码中使用了clone函数而不是pthread_create函数来创建线程,这是因为clone函数可以创建一个新的进程,而线程也是进程的一种,因此可以使用clone函数来创建线程。另外,这段代码中的互斥锁和信号量的使用,可以有效地避免了生产者和消费者之间的竞争和冲突,保证了线程的安全性和正确性。
相关推荐
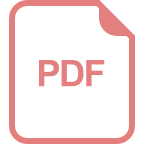
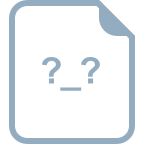
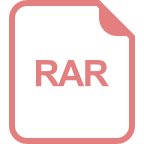
















