在html文件中引入vue组件,并在js文件中控制组件显示隐藏
时间: 2023-08-16 18:04:36 浏览: 120
好的,以下是一个示例代码,演示如何在HTML文件中引入Vue组件,并在JS文件中控制组件的显示和隐藏:
index.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Vue Component Demo</title>
<script src="https://unpkg.com/vue@next"></script>
<!-- 引入 Vue 组件 -->
<script src="./components/MyComponent.js"></script>
</head>
<body>
<div id="app">
<!-- 使用 Vue 组件 -->
<my-component v-if="showComponent"></my-component>
<button @click="toggleComponent">切换显示/隐藏</button>
</div>
<script src="./main.js"></script>
</body>
</html>
```
在上述代码中,我们通过 `<script>` 标签引入了 Vue.js 框架和我们自己编写的 Vue 组件。在 `#app` 元素中,我们使用了 `<my-component>` 标签来使用我们的组件,并使用 `v-if` 指令来控制组件的显示和隐藏。
我们还添加了一个按钮,当用户点击该按钮时,会触发 `toggleComponent` 方法,该方法会切换 `showComponent` 属性的值,从而控制组件的显示和隐藏。
MyComponent.js:
```js
Vue.component('my-component', {
template: `
<div>
<h3>Hello, World!</h3>
</div>
`,
});
export default {
name: 'MyComponent',
};
```
在上述代码中,我们使用 `Vue.component()` 方法注册了一个全局的 Vue 组件。组件的模板中只包含了一个标题,用来展示组件的名称。
main.js:
```js
import { createApp, ref } from 'vue';
import MyComponent from './components/MyComponent.js';
const app = createApp({
components: {
'my-component': MyComponent,
},
setup() {
const showComponent = ref(true);
const toggleComponent = () => {
showComponent.value = !showComponent.value;
};
return {
showComponent,
toggleComponent,
};
},
});
app.mount('#app');
```
在上述代码中,我们使用了 Vue.js 3.x 中的脚手架语法,并通过 `import` 引入了我们的 Vue 组件。在 `createApp()` 方法中,我们将组件注册到了应用中,并在 `setup()` 中定义了 `showComponent` 和 `toggleComponent` 两个变量,用来控制组件的显示和隐藏。
最后,我们通过 `app.mount('#app')` 将应用挂载到 `#app` 元素中。
当用户点击页面中的按钮时,会触发 `toggleComponent` 方法,从而切换 `showComponent` 变量的值。由于我们在 HTML 中使用了 `v-if` 指令,因此组件的显示和隐藏状态会随着 `showComponent` 变量的值而改变。
阅读全文
相关推荐
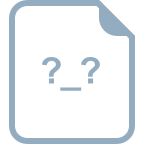
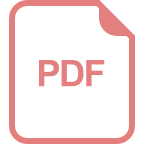
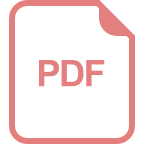
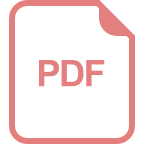
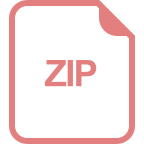
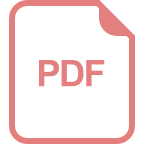
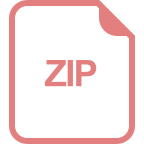
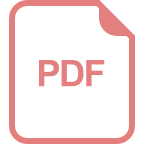
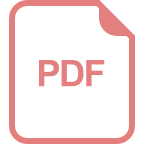
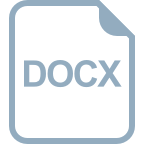
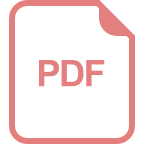
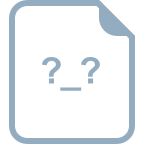
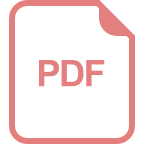
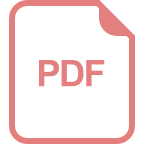