linux串口收发测试程序
时间: 2024-10-15 19:03:33 浏览: 42
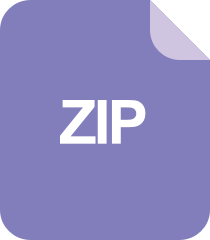
linux串口收发测试程序_ttyUSB_linux_serialport_

在Linux系统中,串口(也称为COM端口或TTY设备)通常用于连接硬件设备如GPS接收器、打印机或其他外设。如果你需要编写一个串口收发测试程序,你可以使用`ncurses`库结合`termios.h`头文件来创建一个用户界面,然后利用`sys/ioctl.h`和`fcntl.h`来操作串口。
以下是一个简单的步骤和示例:
1. **安装依赖**:首先,你需要安装`ncurses`库。在终端中运行 `sudo apt-get install libncurses5-dev` 或者 `yum install ncurses-devel` (取决于你的发行版)。
2. **打开串口**:使用`open()`函数打开串口,设置权限为`O_RDWR`以便读写。例如:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY); // 替换为你的串口号
if (fd == -1) {
perror("Failed to open serial port");
return -1;
}
```
3. **配置串口**:设置波特率、数据位、停止位和校验等串口属性,通常通过`tcgetattr()`和`tcsetattr()`函数:
```c
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600bps
cfsetospeed(&options, B9600);
...
tcflush(fd, TCIOFLUSH); // 清空缓冲区
tcsetattr(fd, TCSANOW, &options);
```
4. **发送和接收数据**:通过`write()`写入数据到串口,`read()`从串口读取数据。确保添加适当的错误处理和数据解析代码。
5. **显示结果**:使用`ncurses`创建用户界面,显示接收到的数据。
以下是一个简化的测试程序结构:
```c
#include <ncurses.h>
void send_data(char *data) {
write(fd, data, strlen(data));
}
void receive_data(char *buffer, int size) {
ssize_t bytes_received = read(fd, buffer, size);
if (bytes_received > 0) {
printf("Received: %s\n", buffer);
}
}
int main() {
initscr(); // 初始化ncurses
// ... 使用ncurses创建窗口和界面 ...
char send_buffer[] = "Hello from Linux!";
char recv_buffer[128];
send_data(send_buffer);
receive_data(recv_buffer, sizeof(recv_buffer));
endwin();
close(fd);
return 0;
}
```
阅读全文
相关推荐

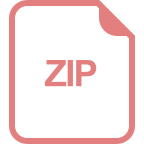
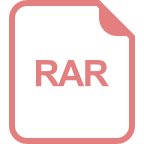
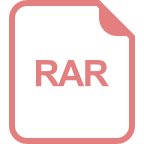

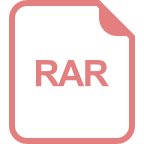
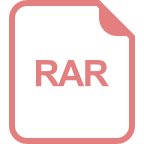
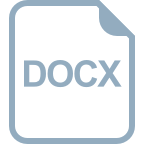
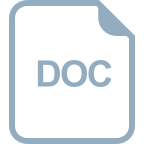
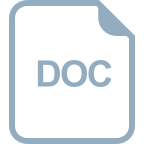
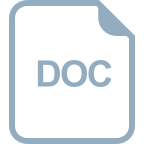
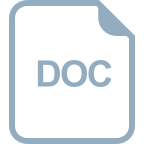
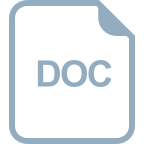

