如何导入import java.text.SimpleDateFormat在eclipse
时间: 2023-11-22 13:50:15 浏览: 72
要在Eclipse中导入`java.text.SimpleDateFormat`类,可以按照以下步骤进行操作:
1. 在Eclipse中打开需要使用`SimpleDateFormat`类的Java源文件。
2. 在源文件的开头,添加`import java.text.SimpleDateFormat;`语句。
3. 如果Eclipse中提示`SimpleDateFormat`类不存在,则需要将JRE库中的Java类库添加到项目的Build Path中。具体操作如下:
a. 右键单击项目名称,选择Properties。
b. 在弹出的Properties对话框中,选择Java Build Path。
c. 在Java Build Path页面的Libraries选项卡中,选择JRE System Library,然后点击Edit。
d. 在弹出的JRE System Library对话框中,选择Workspace default JRE,并点击Finish。
e. 最后点击Apply和OK按钮保存更改。
4. 现在你可以在Java源文件中使用`SimpleDateFormat`类了。
以上是在Eclipse中导入`java.text.SimpleDateFormat`类的简单步骤,希望对你有所帮助。
相关问题
导入import java.text.SimpleDateFormat类,需要在电脑安装嘛?
不需要安装任何软件或库来使用Java内置的SimpleDateFormat类。这个类是Java的标准类库之一,可以直接在Java的开发环境中使用。你只需要在代码中引入java.text包,然后就可以使用SimpleDateFormat类了。例如,可以这样引入:
```java
import java.text.SimpleDateFormat;
```
然后就可以使用SimpleDateFormat类中的方法了。
import java.text.SimpleDateFormat;
这是一个Java的日期格式化类库,可以用于将日期格式化为指定的字符串格式。一般的用法是先创建一个SimpleDateFormat对象,然后调用其format方法将日期格式化为字符串。
示例代码:
```
import java.util.Date;
import java.text.SimpleDateFormat;
public class Example {
public static void main(String[] args) {
Date date = new Date();
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String dateString = formatter.format(date);
System.out.println(dateString);
}
}
```
输出结果为当前时间的字符串格式,例如:2021-06-09 14:30:00。
相关推荐
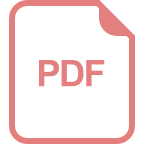
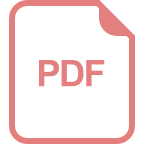
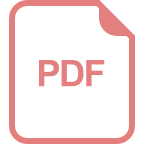













