public class Inventory { static Vector<Goods> goods = new Vector<>(); static Vector<EventO> eventO = new Vector<>(); static Vector<EventR> eventR = new Vector<>(); static Vector<EventD> eventD = new Vector<>(); static Vector<EventA> eventA = new Vector<>(); static Vector<Event> v_shipping = new Vector<>(); static Vector<Event> v_errors = new Vector<>(); public static void main(String[] args) { try { get_Inventory(); //读取库存记录 get_Transactions(); } catch (IOException e) { e.printStackTrace(); //读取事件 } EventA_Handle(); //处理A事件 EventR_Handle(); //处理R事件 EventO_Handle(); //处理O事件 EventD_Handle(); //处理D事件 File_Handle(); //生成shipping、Errors、NewInventory文件 } /** * @description 读取库存记录,存入vector中 */ static void get_Inventory() throws IOException { BufferedReader in = new BufferedReader(new FileReader("src/Inventory.txt")); String line; while((line = in.readLine())!=null) { //按行读取文件 String[] str2 = line.split("\\s+"); //分解字符串 Goods goods_temp = new Goods(str2[0], Integer.parseInt(str2[1]), str2[2], str2[3]); goods.addElement(goods_temp); } in.close(); } /** * @description 读取库存记录,存入vector中 */ static void get_Transactions() throws IOException { //读取事件 BufferedReader in = new BufferedReader(new FileReader("src/Transactions.txt")); String line; while((line = in.readLine())!=null) { //按行读取文件 String[] str2 = line.split("\\s+"); //分解字符串 if (str2[0].equals("O")) { //读取O事件 EventO event_temp = new EventO(str2[1], Integer.parseInt(str2[2]), str2[3]); eventO.addElement(event_temp); } else if (str2[0].equals("R")) { //读取R事件 EventR event_temp = new EventR(str2[1], Integer.parseInt(str2[2])); eventR.addElement(event_temp); } else if (str2[0].equals("D")) { //读取D事件 EventD event_temp = new EventD(str2[1]); eventD.addElement(event_temp); } else if (str2[0].equals("A")) { //读取A事件 EventA event_temp = new EventA(str2[1], str2[2], str2[3]); eventA.addElement(event_temp); } } in.close(); }请为以上代码添加注释
时间: 2023-06-06 17:07:14 浏览: 64
/**
* Inventory类用于处理库存和事件记录相关的操作
*/
public class Inventory {
static Vector<Goods> goods = new Vector<>(); //存放商品记录的向量
static Vector<EventO> eventO = new Vector<>(); //存放O事件记录的向量
static Vector<EventR> eventR = new Vector<>(); //存放R事件记录的向量
static Vector<EventD> eventD = new Vector<>(); //存放D事件记录的向量
static Vector<EventA> eventA = new Vector<>(); //存放A事件记录的向量
static Vector<Event> v_shipping = new Vector<>(); //存放shipping事件记录的向量
static Vector<Event> v_errors = new Vector<>(); //存放Errors事件记录的向量
/**
* 库存及事件记录处理的程序入口
* @param args
*/
public static void main(String[] args) {
try {
get_Inventory(); //读取库存记录
get_Transactions(); //读取事件记录
} catch (IOException e) {
e.printStackTrace();
}
EventA_Handle(); //处理A事件
EventR_Handle(); //处理R事件
EventO_Handle(); //处理O事件
EventD_Handle(); //处理D事件
File_Handle(); //生成shipping、Errors、NewInventory文件
}
/**
* 从文件中读取库存记录,存入商品记录向量中
* @throws IOException
*/
static void get_Inventory() throws IOException {
BufferedReader in = new BufferedReader(new FileReader("src/Inventory.txt"));
String line;
while((line = in.readLine())!=null) { //按行读取文件
String[] str2 = line.split("\\s "); //分解字符串
Goods goods_temp = new Goods(str2[0], Integer.parseInt(str2[1]), str2[2], str2[3]);
goods.addElement(goods_temp);
}
in.close();
}
/**
* 从文件中读取事件记录,分别存入对应的事件记录向量中
* @throws IOException
*/
static void get_Transactions() throws IOException {
BufferedReader in = new BufferedReader(new FileReader("src/Transactions.txt"));
String line;
while((line = in.readLine())!=null) { //按行读取文件
String[] str2 = line.split("\\s "); //分解字符串
if (str2[0].equals("O")) { //读取O事件
EventO event_temp = new EventO(str2[1], Integer.parseInt(str2[2]), str2[3]);
eventO.addElement(event_temp);
} else if (str2[0].equals("R")) { //读取R事件
EventR event_temp = new EventR(str2[1], Integer.parseInt(str2[2]));
eventR.addElement(event_temp);
} else if (str2[0].equals("D")) { //读取D事件
EventD event_temp = new EventD(str2[1]);
eventD.addElement(event_temp);
} else if (str2[0].equals("A")) { //读取A事件
EventA event_temp = new EventA(str2[1], str2[2], str2[3]);
eventA.addElement(event_temp);
}
}
in.close();
}
}
相关推荐
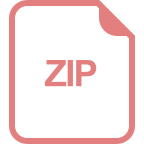
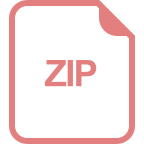


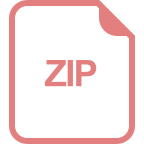
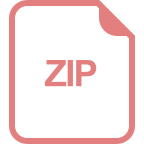
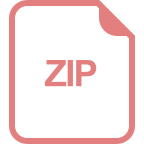
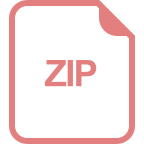
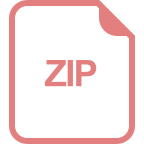
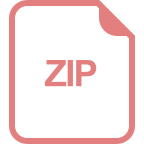
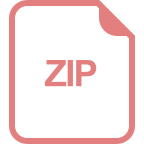