Linux写文本c语言程序完整
时间: 2024-11-09 10:17:50 浏览: 19
在Linux环境下编写C语言程序用于处理文本,通常包括以下几个步骤:
1. **创建新文件**:
打开终端,使用`nano`, `vim` 或 `gedit`等文本编辑器创建一个新的C源文件,例如`textprocessor.c`。
```sh
$ nano textprocessor.c
```
2. **编写代码**:
使用C语法编写代码,比如读取文件、操作字符串、写入文件等。例如,你可以使用`fopen`打开文件,`fgets`读取行,`strstr`查找特定内容,然后使用`fprintf`写回结果。
```c
#include <stdio.h>
#include <string.h>
int main() {
FILE *file;
char line[100], search_str[] = "Hello";
// 打开文件(如果不存在则创建)
file = fopen("input.txt", "r+");
if (file == NULL) {
printf("Error opening the file.\n");
return 1;
}
while (fgets(line, sizeof(line), file)) {
if (strstr(line, search_str)) {
printf("Found '%s' in line: %s\n", search_str, line);
}
}
// 关闭文件
fclose(file);
return 0;
}
```
这里我们搜索了一个名为"input.txt"的文件,如果找到"Hello"就会打印出来。
3. **编译和运行**:
使用`gcc` 编译器编译你的程序,并通过`./a.out`来运行它,这里的`a.out`是默认的可执行文件名,实际使用时需要替换为你编译后的文件名。
```sh
$ gcc -o a.out textprocessor.c
$ ./a.out
```
4. **错误检查**:
程序完成后,记得检查是否有语法错误或者逻辑错误,可以通过查看控制台输出或日志文件来找出问题。
阅读全文
相关推荐
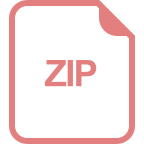
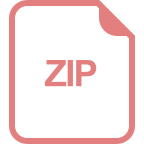
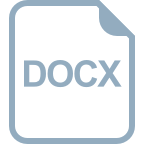
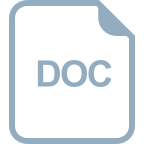
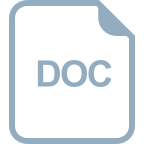
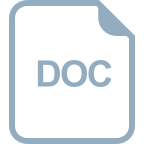
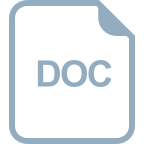










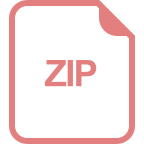
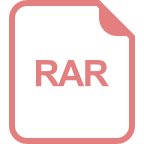