'R0402'如何通过python用正则表达式写出来,并且打印matchObj.group(1)时为r,打印matchObj.group(2)时为R0402
时间: 2024-02-16 07:04:09 浏览: 66
可以使用如下的正则表达式来匹配'R0402':
```python
import re
text = "rR0402 Resistor"
pattern = r'([rR])(\d{4})'
match = re.search(pattern, text)
if match:
print(match.group(1)) # 打印r
print(match.group(2)) # 打印R0402
else:
print("No match found")
```
该正则表达式中,`([rR])`表示匹配字符`r`或`R`,括号表示分组,`(\d{4})`表示匹配任意四个数字,同样使用了括号进行分组。两个括号分别对应两个分组,可以通过`matchObj.group(1)`和`matchObj.group(2)`获取对应的结果。
相关问题
compile正则表达式
编译正则表达式是将正则表达式转换成一种特定的内部表示形式,以便于后续的匹配操作。在 Python 中,我们可以使用 `re.compile()` 函数来编译正则表达式。
下面是一个例子,演示了如何使用 `re.compile()` 函数编译一个简单的正则表达式,并用它来匹配字符串:
```python
import re
# 编译正则表达式
pattern = re.compile(r'\d+')
# 匹配字符串
match = pattern.search('hello123world456')
# 输出匹配结果
if match:
print(match.group())
```
在上面的代码中,我们首先使用 `re.compile()` 函数将正则表达式 `\d+` 编译成一个正则对象 `pattern`,然后使用 `pattern.search()` 方法在字符串 `'hello123world456'` 中搜索匹配项。最后,我们输出匹配结果 `123`。
需要注意的是,使用正则对象进行匹配的方法与使用 `re` 模块的函数是相同的,比如 `pattern.search()` 方法与 `re.search()` 函数的功能是完全一致的。不同之处在于,使用正则对象进行匹配可以提高效率,特别是在需要多次匹配同一个正则表达式时。
使用python中的正则表达式例化verilog代码
可以使用Python中的re模块来实现对Verilog代码的正则表达式匹配和提取。下面是一个例子,演示了如何使用正则表达式匹配Verilog代码中的模块声明和端口声明:
```python
import re
# Verilog代码
code = '''
module my_module (
input clk,
input rst,
input [7:0] in_data,
output [7:0] out_data
);
// 模块实现
...
endmodule
'''
# 匹配模块名称和端口声明
pattern = r"module\s+(\w+)\s*\((.*?)\);"
# 提取模块名称和端口名称
match = re.search(pattern, code, re.DOTALL)
if match:
module_name = match.group(1)
port_list = match.group(2)
# 匹配端口名称和方向
port_pattern = r"\b(input|output|inout)\s+(.*?)\s*,?"
ports = re.findall(port_pattern, port_list)
# 输出结果
print("Module name: ", module_name)
print("Ports: ")
for direction, name in ports:
print(direction, name)
```
上述代码首先定义了一个Verilog代码字符串,然后使用正则表达式模式匹配模块名称和端口声明。接着,通过re.search函数检查匹配是否成功,并使用group方法提取模块名称和端口列表。最后,使用正则表达式模式匹配端口名称和方向,并使用re.findall函数提取所有匹配项。输出结果包括模块名称和端口列表。
阅读全文
相关推荐
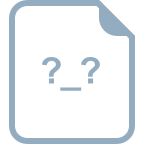
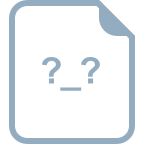











