对英文文本单词统计并对单词次数进行降序排序的java代码怎么写
时间: 2024-01-18 07:01:59 浏览: 65
以下是一个简单的Java程序,可以对输入的文本进行单词统计并按照单词出现次数进行降序排序:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
// 读取输入文本
Scanner scanner = new Scanner(System.in);
String text = scanner.nextLine();
// 将文本转换为单词数组
String[] words = text.toLowerCase().split(" ");
// 统计单词出现次数
Map<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
// 对单词按照出现次数进行降序排序
List<Map.Entry<String, Integer>> sortedWords = new ArrayList<>(wordCount.entrySet());
Collections.sort(sortedWords, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o2.getValue().compareTo(o1.getValue());
}
});
// 输出结果
for (Map.Entry<String, Integer> entry : sortedWords) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
}
}
```
这个程序使用了Java的Map和List集合来实现单词统计和排序。其中,Map用于存储单词出现次数,List用于对单词按照出现次数进行排序。在排序时,我们使用了Java的Comparator接口来定义排序规则。最后,程序将排序后的结果输出到控制台。
相关推荐
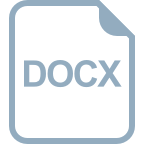
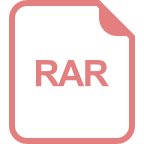









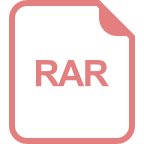
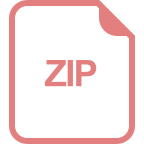