请给出同学录管理系统的c语言代码 功能要求: (1)、程序启动后显示主菜单,包括下面的选项:1.显示班级列表 2.增加班级 3.删除班级 4、班级同学加入 5.班级同学删除 6.同学录显示 7.同学录存盘 8.同学录文件调入 9.退出 (2)按下相应的按键后进入各自的子功能,每个子功能执行完毕后,返团并显示主菜单 功能说明: ①,显示班级列表:在屏幕显示已登记的班级总数,及各班级名称及加入同学人数。② 增加班级:输入班级信息,包括班号,入学年份,毕业年份。③.删除班级:输入要删除的班号,删除该班级信息。 ④班级同学加入:输入同学姓名,性别,班号,学号。 ⑤.班级同学删除:输入班名,学号,删除同学信息,⑥、同学录显示:输入班号,显示该班的同学列表, ⑦.同学录存盘:输入文件名,将同学录信息存入文件。 ⑧.同学录文件调入:输入文件名,读取文件将数据调入,重新生成同学录信息⑨.退出:退出程序,返回 操作系统
时间: 2024-02-12 18:03:57 浏览: 145
好的,根据您提供的功能要求,以下是同学录管理系统的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CLASS_NUM 10
#define MAX_STUDENT_NUM 100
struct Student {
char name[20];
char gender[3];
char class_id[10];
char student_id[20];
};
struct Class {
char id[10];
char enter_year[5];
char graduate_year[5];
int student_num;
struct Student students[MAX_STUDENT_NUM];
};
struct Class classes[MAX_CLASS_NUM];
int class_num = 0;
void show_menu();
void show_class_list();
void add_class();
void delete_class();
void add_student();
void delete_student();
void show_student_list();
void save_to_file();
void load_from_file();
int main() {
while (1) {
show_menu();
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
show_class_list();
break;
case 2:
add_class();
break;
case 3:
delete_class();
break;
case 4:
add_student();
break;
case 5:
delete_student();
break;
case 6:
show_student_list();
break;
case 7:
save_to_file();
break;
case 8:
load_from_file();
break;
case 9:
printf("Goodbye!\n");
exit(0);
default:
printf("Invalid input, please try again.\n");
}
}
}
void show_menu() {
printf("Please select an option:\n");
printf("1. Show class list\n");
printf("2. Add class\n");
printf("3. Delete class\n");
printf("4. Add student\n");
printf("5. Delete student\n");
printf("6. Show student list\n");
printf("7. Save to file\n");
printf("8. Load from file\n");
printf("9. Quit\n");
}
void show_class_list() {
printf("Class number: %d\n", class_num);
printf("Class ID\tEnter year\tGraduate year\tStudent number\n");
for (int i = 0; i < class_num; i++) {
printf("%s\t\t%s\t\t%s\t\t%d\n", classes[i].id, classes[i].enter_year, classes[i].graduate_year, classes[i].student_num);
}
}
void add_class() {
if (class_num >= MAX_CLASS_NUM) {
printf("Class number has reached the limit.\n");
return;
}
printf("Please enter the class ID: ");
scanf("%s", classes[class_num].id);
printf("Please enter the enter year: ");
scanf("%s", classes[class_num].enter_year);
printf("Please enter the graduate year: ");
scanf("%s", classes[class_num].graduate_year);
classes[class_num].student_num = 0;
class_num++;
}
void delete_class() {
char id[10];
printf("Please enter the class ID: ");
scanf("%s", id);
int index = -1;
for (int i = 0; i < class_num; i++) {
if (strcmp(classes[i].id, id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Class not found.\n");
return;
}
for (int i = index; i < class_num - 1; i++) {
classes[i] = classes[i + 1];
}
class_num--;
printf("Class deleted.\n");
}
void add_student() {
char class_id[10];
printf("Please enter the class ID: ");
scanf("%s", class_id);
int index = -1;
for (int i = 0; i < class_num; i++) {
if (strcmp(classes[i].id, class_id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Class not found.\n");
return;
}
if (classes[index].student_num >= MAX_STUDENT_NUM) {
printf("Student number has reached the limit.\n");
return;
}
printf("Please enter the student name: ");
scanf("%s", classes[index].students[classes[index].student_num].name);
printf("Please enter the student gender: ");
scanf("%s", classes[index].students[classes[index].student_num].gender);
printf("Please enter the student ID: ");
scanf("%s", classes[index].students[classes[index].student_num].student_id);
strcpy(classes[index].students[classes[index].student_num].class_id, class_id);
classes[index].student_num++;
}
void delete_student() {
char class_id[10];
char student_id[20];
printf("Please enter the class ID: ");
scanf("%s", class_id);
printf("Please enter the student ID: ");
scanf("%s", student_id);
int class_index = -1;
int student_index = -1;
for (int i = 0; i < class_num; i++) {
if (strcmp(classes[i].id, class_id) == 0) {
class_index = i;
break;
}
}
if (class_index == -1) {
printf("Class not found.\n");
return;
}
for (int i = 0; i < classes[class_index].student_num; i++) {
if (strcmp(classes[class_index].students[i].student_id, student_id) == 0) {
student_index = i;
break;
}
}
if (student_index == -1) {
printf("Student not found.\n");
return;
}
for (int i = student_index; i < classes[class_index].student_num - 1; i++) {
classes[class_index].students[i] = classes[class_index].students[i + 1];
}
classes[class_index].student_num--;
printf("Student deleted.\n");
}
void show_student_list() {
char class_id[10];
printf("Please enter the class ID: ");
scanf("%s", class_id);
int index = -1;
for (int i = 0; i < class_num; i++) {
if (strcmp(classes[i].id, class_id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("Class not found.\n");
return;
}
printf("Student number: %d\n", classes[index].student_num);
printf("Student name\tGender\tStudent ID\n");
for (int i = 0; i < classes[index].student_num; i++) {
printf("%s\t\t%s\t%s\n", classes[index].students[i].name, classes[index].students[i].gender, classes[index].students[i].student_id);
}
}
void save_to_file() {
char filename[20];
printf("Please enter the file name: ");
scanf("%s", filename);
FILE *fp;
fp = fopen(filename, "wb");
if (fp == NULL) {
printf("Failed to open file.\n");
return;
}
fwrite(&class_num, sizeof(int), 1, fp);
fwrite(classes, sizeof(struct Class), class_num, fp);
fclose(fp);
printf("Data saved.\n");
}
void load_from_file() {
char filename[20];
printf("Please enter the file name: ");
scanf("%s", filename);
FILE *fp;
fp = fopen(filename, "rb");
if (fp == NULL) {
printf("Failed to open file.\n");
return;
}
fread(&class_num, sizeof(int), 1, fp);
fread(classes, sizeof(struct Class), class_num, fp);
fclose(fp);
printf("Data loaded.\n");
}
```
注意:以上代码仅为示例,可能存在不足之处,需要根据实际情况进行调整和完善。
阅读全文
相关推荐
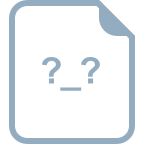
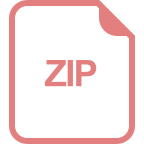
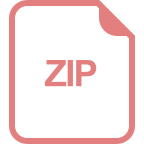















