用html,css和js写一个俄罗斯方块游戏
时间: 2023-03-19 18:24:59 浏览: 84
俄罗斯方块游戏是一款经典的游戏,可以使用 HTML、CSS 和 JavaScript 来实现。
下面是一个简单的俄罗斯方块游戏的示例代码,仅供参考:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>俄罗斯方块游戏</title>
<style>
/* CSS 样式表 */
#game-board {
width: 300px;
height: 600px;
border: 1px solid black;
margin: 0 auto;
}
.game-cell {
width: 30px;
height: 30px;
border: 1px solid black;
background-color: #eee;
float: left;
}
.active {
background-color: #00f;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script src="tetris.js"></script>
</body>
</html>
```
CSS 样式表定义了游戏板和游戏方块的样式。
JavaScript 代码:
```javascript
// JavaScript 代码
const ROWS = 20;
const COLS = 10;
const board = [];
for (let row = 0; row < ROWS; row++) {
board[row] = [];
for (let col = 0; col < COLS; col++) {
board[row][col] = 0;
}
}
const tetrominos = [
[0x0f00, 0x2222, 0x00f0, 0x4444],
[0x44c0, 0x8e00, 0x6440, 0x0e20],
[0x4460, 0x0e80, 0xc440, 0x2e00],
[0xcc00, 0xcc00, 0xcc00, 0xcc00],
[0x06c0, 0x8c40, 0x6c00, 0x4620],
[0x0e40, 0x4c40, 0x4e00, 0x4640],
[0x0c60, 0x4c80, 0xc600, 0x2640],
];
let currentTetromino = getRandomTetromino();
let currentX = 4;
let currentY = 0;
function getRandomTetromino() {
const tetrominoIndex = Math.floor(Math.random() * tetrominos.length);
return tetrominos[tetrominoIndex];
}
function rotateTetromino(tetromino) {
const rotatedTetromino = [];
for (let row = 0; row < 4; row++) {
rotatedTetromino[row] = 0;
for (let col = 0; col < 4; col++) {
rotatedTetromino[row] |= ((tetromino[col] >> (3 - row) * 4) & 0xf) << (3 - col) * 4;
}
}
return rotatedTetromino;
}
function drawBoard() {
const gameBoard = document.getElementById('game-board');
gameBoard.innerHTML = '';
for (let row = 0; row < ROWS;
相关推荐
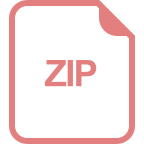
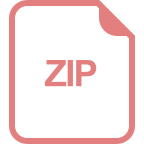
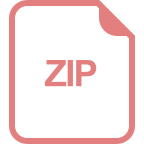









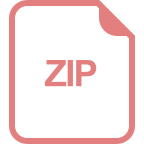
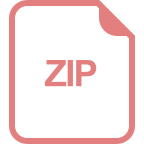
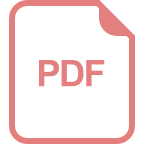
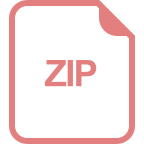
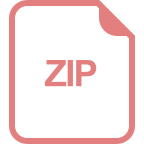