golang iris mvc实现表增删改查源代码,包含router/model/service/controller/config
时间: 2023-10-09 13:07:07 浏览: 203
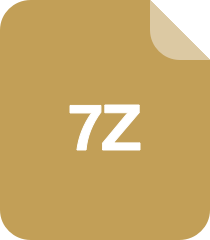
mvc实现增删改查,初学可以参考一下下

很高兴回答这个问题。以下是一个基于Go语言Iris框架的MVC(模型-视图-控制器)架构的源代码实现:
1、router.go:
```
package router
import (
"github.com/kataras/iris/v12"
"myproject/controller"
)
func Router(app *iris.Application) {
userController := controller.UserController{}
app.Handle("GET", "/users", userController.GetAll)
app.Handle("GET", "/user/{id}", userController.GetByID)
app.Handle("POST", "/user", userController.Create)
app.Handle("PUT", "/user/{id}", userController.UpdateByID)
app.Handle("DELETE", "/user/{id}", userController.DeleteByID)
}
```
2、model.go:
```
package model
import (
"gorm.io/driver/mysql"
"gorm.io/gorm"
"myproject/config"
)
var (
DB *gorm.DB
)
func Init() {
dsn := config.GetDSN()
var err error
DB, err = gorm.Open(mysql.Open(dsn), &gorm.Config{})
if err != nil {
panic(err)
}
DB.AutoMigrate(&User{})
}
type User struct {
gorm.Model
Name string `gorm:"type:varchar(32)"`
Age int `gorm:"default:18"`
}
func (u *User) TableName() string {
return "users"
}
```
3、service.go:
```
package service
import (
"myproject/model"
)
type UserService struct{}
func (us *UserService) GetAll() []*model.User {
var users []*model.User
model.DB.Find(&users)
return users
}
func (us *UserService) GetByID(id int) *model.User {
user := &model.User{}
model.DB.First(user, id)
return user
}
func (us *UserService) Create(user *model.User) {
model.DB.Create(user)
}
func (us *UserService) UpdateByID(id int, user *model.User) {
model.DB.Model(&model.User{}).Where("id=?", id).Updates(&user)
}
func (us *UserService) DeleteByID(id int) {
model.DB.Delete(&model.User{}, id)
}
```
4、controller.go:
```
package controller
import (
"github.com/kataras/iris/v12"
"myproject/model"
"myproject/service"
"strconv"
)
type UserController struct{}
func (uc *UserController) GetAll(ctx iris.Context) {
userService := &service.UserService{}
result := userService.GetAll()
ctx.JSON(result)
}
func (uc *UserController) GetByID(ctx iris.Context) {
userService := &service.UserService{}
id, _ := strconv.Atoi(ctx.Params().Get("id"))
result := userService.GetByID(id)
ctx.JSON(result)
}
func (uc *UserController) Create(ctx iris.Context) {
userService := &service.UserService{}
user := &model.User{}
ctx.ReadJSON(&user)
userService.Create(user)
}
func (uc *UserController) UpdateByID(ctx iris.Context) {
userService := &service.UserService{}
user := &model.User{}
id, _ := strconv.Atoi(ctx.Params().Get("id"))
ctx.ReadJSON(&user)
userService.UpdateByID(id, user)
}
func (uc *UserController) DeleteByID(ctx iris.Context) {
userService := &service.UserService{}
id, _ := strconv.Atoi(ctx.Params().Get("id"))
userService.DeleteByID(id)
}
```
5、config.go:
```
package config
func GetDSN() string {
return "root:123456@tcp(127.0.0.1:3306)/mydb?charset=utf8mb4&parseTime=True&loc=Local"
}
```
这是一份基础的代码实现,你可以根据你的需求进行相应的修改和优化。希望对你有帮助。
阅读全文
相关推荐
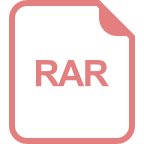
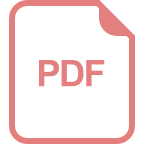
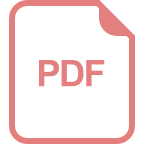
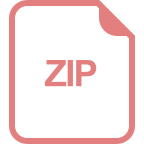
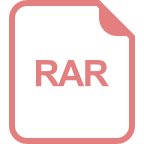
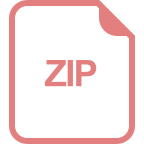
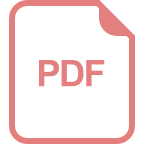
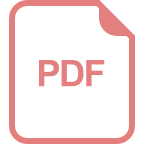
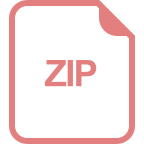
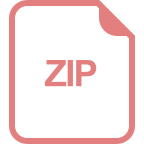
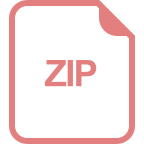
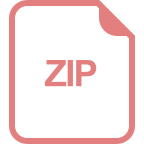
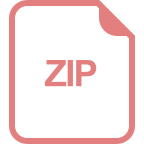