java 图片自适应按钮大小且使得图片透明的区域为透明
时间: 2024-02-28 10:54:47 浏览: 59
要实现图片自适应按钮大小且使得图片透明的区域为透明,可以使用Java的Swing库中的JButton和ImageIcon类。
首先创建一个JButton对象,然后使用ImageIcon类将图片加载到JButton上。接着可以使用getPreferredSize()方法获取图片的实际大小,然后将JButton的大小设置为图片的大小,从而实现图片自适应按钮大小的效果。
为了使得图片透明的区域为透明,需要对图片进行处理。可以使用Java的BufferedImage类,将图片转换为可编辑的BufferedImage对象。然后遍历所有像素,将图片中透明的部分的像素的Alpha值设置为0,从而使得透明的区域为透明。
下面是一个示例代码:
```
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
public class TransparentButton extends JButton {
private BufferedImage image;
public TransparentButton(String imagePath) {
try {
// Load the image as a BufferedImage
image = ImageIO.read(new File(imagePath));
// Make the button the same size as the image
setPreferredSize(new Dimension(image.getWidth(), image.getHeight()));
// Make the transparent parts of the image transparent in the button
makeTransparent();
} catch (IOException e) {
e.printStackTrace();
}
}
private void makeTransparent() {
// Create a new BufferedImage that is compatible with the current screen
BufferedImage bi = new BufferedImage(image.getWidth(), image.getHeight(), BufferedImage.TYPE_INT_ARGB);
// Draw the image onto the new BufferedImage
Graphics g = bi.getGraphics();
g.drawImage(image, 0, 0, null);
g.dispose();
// Loop through all the pixels in the image
for (int x = 0; x < bi.getWidth(); x++) {
for (int y = 0; y < bi.getHeight(); y++) {
// Get the color of the pixel
Color c = new Color(bi.getRGB(x, y), true);
// If the alpha component of the color is 0 (transparent), set the alpha component to 0
if (c.getAlpha() == 0) {
c = new Color(c.getRed(), c.getGreen(), c.getBlue(), 0);
bi.setRGB(x, y, c.getRGB());
}
}
}
// Set the button's icon to the new BufferedImage
setIcon(new ImageIcon(bi));
}
public static void main(String[] args) {
JFrame frame = new JFrame("Transparent Button Test");
TransparentButton button = new TransparentButton("image.png");
frame.getContentPane().add(button);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
在上面的代码中,我们创建了一个继承自JButton的类TransparentButton,它从文件系统中加载了一张图片,将图片透明的区域处理为透明,并将处理后的图片设置为按钮的图标。在main()方法中,我们创建了一个JFrame,并将TransparentButton添加到JFrame中。当运行程序时,我们会看到一个自适应大小的按钮,其中图片的透明部分是透明的。
阅读全文
相关推荐
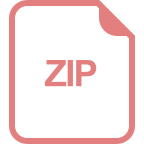
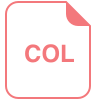
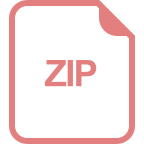
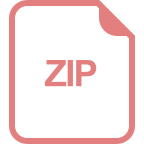
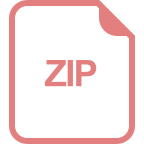
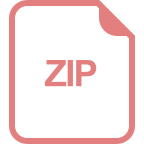