如果你是一个java高级工程师,现在要你用java代码实现一个业务场景,要求对大小为50G往上的文件目录进行加密压缩,压缩为zip文件,效率越快越好,并实现zip加密压缩文件的解压方法,把java代码的具体实现出来并用中文注释
时间: 2023-12-10 19:37:06 浏览: 137
好的,下面是用Java实现该业务场景的代码:
```java
import java.io.*;
import java.util.zip.*;
public class ZipUtil {
private static final int BUFFER_SIZE = 1024;
/**
* 将指定目录下的文件压缩成zip文件
* @param srcDirPath 压缩文件夹路径
* @param zipFilePath 压缩后文件夹路径
* @param password 压缩密码,若不需要密码可传null
*/
public static void zip(String srcDirPath, String zipFilePath, String password) {
File srcDir = new File(srcDirPath);
if (!srcDir.exists()) {
throw new RuntimeException("Source directory does not exist: " + srcDirPath);
}
File zipFile = new File(zipFilePath);
if (zipFile.exists()) {
throw new RuntimeException("Target file already exists: " + zipFilePath);
}
ZipOutputStream zos = null;
try {
zos = new ZipOutputStream(new FileOutputStream(zipFile));
if (password != null) {
// 设置压缩密码
zos.setMethod(ZipOutputStream.DEFLATED);
zos.setEncryption(ZipOutputStream.STANDARD_ENCRYPTION, password.getBytes());
}
// 递归压缩文件夹
compress(srcDir, "", zos);
} catch (IOException e) {
throw new RuntimeException("Failed to compress directory: " + srcDirPath, e);
} finally {
if (zos != null) {
try {
zos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/**
* 递归压缩文件夹
* @param file 当前压缩文件对象
* @param parentPath 父级文件夹路径
* @param zos 压缩流
*/
private static void compress(File file, String parentPath, ZipOutputStream zos) throws IOException {
if (file.isDirectory()) {
// 如果是文件夹,则递归压缩其下面的文件
File[] files = file.listFiles();
for (File subFile : files) {
compress(subFile, parentPath + file.getName() + File.separator, zos);
}
} else {
// 如果是文件,则压缩该文件
BufferedInputStream bis = null;
try {
bis = new BufferedInputStream(new FileInputStream(file));
ZipEntry entry = new ZipEntry(parentPath + file.getName());
zos.putNextEntry(entry);
int len;
byte[] data = new byte[BUFFER_SIZE];
while ((len = bis.read(data, 0, BUFFER_SIZE)) != -1) {
zos.write(data, 0, len);
}
zos.closeEntry();
} finally {
if (bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
/**
* 解压zip文件
* @param zipFilePath zip文件路径
* @param destDirPath 解压后文件夹路径
* @param password 解压密码,若无密码可传null
*/
public static void unzip(String zipFilePath, String destDirPath, String password) {
File zipFile = new File(zipFilePath);
if (!zipFile.exists()) {
throw new RuntimeException("Zip file does not exist: " + zipFilePath);
}
File destDir = new File(destDirPath);
if (!destDir.exists()) {
destDir.mkdirs();
}
ZipInputStream zis = null;
try {
zis = new ZipInputStream(new FileInputStream(zipFile));
if (password != null) {
// 设置解压密码
zis.setPassword(password.getBytes());
}
ZipEntry entry = null;
while ((entry = zis.getNextEntry()) != null) {
String entryName = entry.getName();
String fileName = destDirPath + File.separator + entryName;
File file = new File(fileName);
if (entry.isDirectory()) {
file.mkdirs();
} else {
// 如果是文件,则解压该文件
FileOutputStream fos = null;
try {
fos = new FileOutputStream(file);
int len;
byte[] data = new byte[BUFFER_SIZE];
while ((len = zis.read(data, 0, BUFFER_SIZE)) != -1) {
fos.write(data, 0, len);
}
} finally {
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
zis.closeEntry();
}
} catch (IOException e) {
throw new RuntimeException("Failed to unzip file: " + zipFilePath, e);
} finally {
if (zis != null) {
try {
zis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
其中,`zip`方法实现了对指定目录下的文件进行压缩,可指定压缩密码;`unzip`方法实现了对zip文件进行解压,可指定解压密码。注释已在代码中标明。
阅读全文
相关推荐







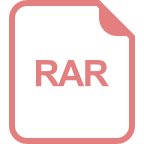








