resttemplate.exchange get传参方式 代码示例
时间: 2023-08-31 19:30:20 浏览: 244
### 回答1:
使用RestTemplate的exchange方法实现GET请求并传递参数的代码示例如下:
```
RestTemplate restTemplate = new RestTemplate();
String url = "http://example.com/api/users?id={id}";
Map<String, String> params = new HashMap<>();
params.put("id", "123");
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class, params);
```
在这个代码示例中,我们首先创建了一个RestTemplate对象,然后构造了一个请求URL,其中{id}是一个占位符,表示我们将要传递的参数。然后我们创建了一个Map,并在其中放入了一个名为"id"的键和对应的参数值"123"。最后,我们使用exchange方法发送GET请求,并将请求结果赋值给ResponseEntity对象。
### 回答2:
resttemplate.exchange方法是Spring提供的一个HTTP请求发送的工具方法。在使用get请求时,可以通过以下方式传递参数。
首先,创建一个HttpHeaders对象,用于设置请求头信息。例如:
```
HttpHeaders headers = new HttpHeaders();
headers.add("User-Agent", "Mozilla/5.0");
```
然后,创建一个HttpEntity对象,用于设置请求体信息和请求头信息。例如:
```
HttpEntity<String> requestEntity = new HttpEntity<>(headers);
```
接下来,使用resttemplate.exchange方法发送get请求,并传入请求URL,请求方式,请求头和返回值类型。例如:
```
String url = "http://api.example.com/users";
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, requestEntity, String.class);
```
最后,通过response对象获取请求的结果,如状态码、响应头和响应体。例如:
```
HttpStatus statusCode = response.getStatusCode();
HttpHeaders responseHeaders = response.getHeaders();
String responseBody = response.getBody();
```
以上就是使用resttemplate.exchange方法进行get请求传参的代码示例。在示例中,我们通过设置请求头信息和请求体信息,然后使用exchange方法发送get请求,并获取请求的结果。
### 回答3:
RestTemplate是Spring框架提供的一个用于发送HTTP请求的类,可以方便地进行GET请求并传递参数。在使用RestTemplate发送GET请求传递参数的方式主要有两种,一种是使用普通参数传递,另一种是使用URI模板传递参数。
1. 使用普通参数传递:
示例代码如下:
```java
// 创建RestTemplate对象
RestTemplate restTemplate = new RestTemplate();
// 设置请求参数
String url = "http://example.com/api?param1=value1¶m2=value2";
// 发送GET请求并接收响应
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class);
// 获取响应结果
String result = response.getBody();
```
在上述代码中,我们通过在url中使用"?"来指定参数的起始位置,然后使用"&"来分隔多个参数,并使用"="来连接参数名和参数值。通过这种方式,可以一次性传递多个参数。
2. 使用URI模板传递:
示例代码如下:
```java
// 创建RestTemplate对象
RestTemplate restTemplate = new RestTemplate();
// 设置请求参数
String url = "http://example.com/api/{param1}/{param2}";
// 设置URI参数值
Map<String, String> params = new HashMap<>();
params.put("param1", "value1");
params.put("param2", "value2");
// 发送GET请求并接收响应
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, null, String.class, params);
// 获取响应结果
String result = response.getBody();
```
在以上代码中,我们使用"{param}"的形式来表示模板参数,在url中使用对应的参数名称,然后在exchange方法的参数列表中传递一个Map对象,其中key为参数名称,value为参数值。RestTemplate会自动将Map的参数值替换到URL对应的位置上,完成参数传递。
总结起来,RestTemplate.exchange方法可以用于发送GET请求并传递参数,可以使用普通参数传递或者使用URI模板传递参数,使用起来非常方便。
阅读全文
相关推荐
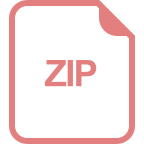
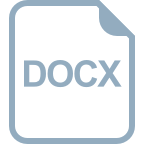















