java applet 和 Graphics2D 绘制动态太极图
时间: 2024-04-29 09:22:07 浏览: 103
好的,我可以为您提供一些基本的代码来绘制动态太极图。首先,我们需要创建一个继承自 Applet 类的 Java 类,然后在该类中实现 paint() 方法。在 paint() 方法中,我们将使用 Graphics2D 对象来绘制太极图形。
以下代码演示了如何使用 Graphics2D 绘制一个简单的太极图形:
```
import java.applet.Applet;
import java.awt.*;
public class TaiChi extends Applet implements Runnable {
private Thread thread;
private Graphics2D g2d;
private int centerX, centerY;
private int radius;
public void init() {
setSize(400, 400);
setBackground(Color.WHITE);
centerX = getWidth() / 2;
centerY = getHeight() / 2;
radius = Math.min(getWidth(), getHeight()) / 2;
}
public void start() {
thread = new Thread(this);
thread.start();
}
public void stop() {
thread = null;
}
public void run() {
while (thread != null) {
repaint();
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void paint(Graphics g) {
g2d = (Graphics2D) g;
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setColor(Color.BLACK);
g2d.fillOval(centerX - radius, centerY - radius, radius * 2, radius * 2);
g2d.setColor(Color.WHITE);
g2d.fillArc(centerX - radius, centerY - radius, radius * 2, radius * 2, 0, 180);
g2d.fillArc(centerX - radius, centerY - radius, radius * 2, radius * 2, 180, 180);
g2d.setColor(Color.BLACK);
g2d.drawOval(centerX - radius, centerY - radius, radius * 2, radius * 2);
g2d.setColor(Color.WHITE);
g2d.fillOval(centerX - radius / 2, centerY - radius / 2, radius, radius);
g2d.setColor(Color.BLACK);
g2d.drawOval(centerX - radius / 2, centerY - radius / 2, radius, radius);
g2d.setColor(Color.WHITE);
g2d.fillArc(centerX - radius / 2, centerY, radius, radius, 0, 180);
g2d.setColor(Color.BLACK);
g2d.drawArc(centerX - radius / 2, centerY, radius, radius, 0, 180);
g2d.setColor(Color.BLACK);
g2d.fillArc(centerX - radius / 2, centerY - radius, radius, radius, 0, -180);
g2d.setColor(Color.WHITE);
g2d.drawArc(centerX - radius / 2, centerY - radius, radius, radius, 0, -180);
}
}
```
在上述代码中,我们使用了 Graphics2D 对象来绘制太极图形。首先,我们设置了渲染提示来开启反锯齿功能。然后,我们使用 fillOval() 方法绘制了黑色的圆形。接下来,我们使用 fillArc() 方法绘制了两个白色的半圆形,并将它们分别放置在圆形的上半部分和下半部分。最后,我们使用 fillOval() 和 fillArc() 方法绘制了太极图形中的小圆和两个小半圆。
在 init() 方法中,我们计算了中心点和半径的值,以便在 paint() 方法中使用。在 start() 方法中,我们启动了一个线程来定期调用 repaint() 方法,从而更新太极图形。在 paint() 方法中,我们首先获取 Graphics2D 对象并设置了渲染提示,然后绘制了太极图形的各个部分。
运行上述代码,您将看到一个简单的太极图形在 Applet 窗口中动态绘制。您可以尝试修改代码来实现更复杂的动态效果。
阅读全文
相关推荐





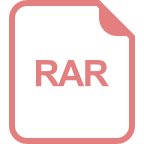
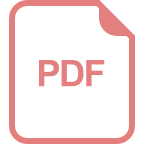




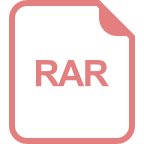
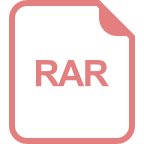
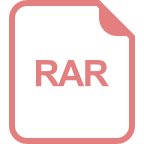


