jsch使用RSA证书例子
时间: 2023-07-11 10:22:18 浏览: 112
下面是一个使用JSch库进行SSH连接并使用RSA证书进行身份验证的例子:
```java
import com.jcraft.jsch.*;
public class SSHConnection {
public static void main(String[] args) {
JSch jsch = new JSch();
Session session = null;
try {
// 设置私钥文件路径
jsch.addIdentity("/path/to/private.key");
// 创建SSH连接
session = jsch.getSession("username", "remote.host.com", 22);
// 设置StrictHostKeyChecking属性为no,避免第一次连接时询问是否连接
session.setConfig("StrictHostKeyChecking", "no");
// 连接SSH服务器
session.connect();
// 执行命令
Channel channel = session.openChannel("exec");
((ChannelExec) channel).setCommand("ls -l");
channel.setInputStream(null);
((ChannelExec) channel).setErrStream(System.err);
InputStream in = channel.getInputStream();
channel.connect();
byte[] tmp = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(tmp, 0, 1024);
if (i < 0) break;
System.out.print(new String(tmp, 0, i));
}
if (channel.isClosed()) {
if (in.available() > 0) continue;
System.out.println("exit-status: " + channel.getExitStatus());
break;
}
try {
Thread.sleep(1000);
} catch (Exception ee) {
}
}
channel.disconnect();
session.disconnect();
} catch (JSchException | IOException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,我们使用`jsch.addIdentity()`方法将私钥文件添加到JSch对象中,然后使用`jsch.getSession()`方法创建一个SSH连接对象。在连接对象中,我们可以设置连接的一些属性,例如`StrictHostKeyChecking`属性,表示是否进行主机密钥检查。
最后,我们通过`session.connect()`方法连接SSH服务器,然后可以使用`Channel`对象执行命令或传输文件等操作。需要注意的是,在使用完后,我们需要调用`channel.disconnect()`和`session.disconnect()`方法关闭连接。
希望这个例子对你有所帮助。
阅读全文
相关推荐
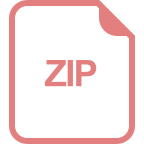






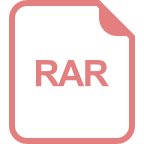
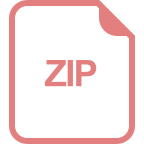







