tcp header结构体组成
时间: 2023-09-18 10:08:23 浏览: 164
TCP头部结构体主要由以下字段组成:
- 源端口号(Source Port):16位,用于标识发送端口号。
- 目标端口号(Destination Port):16位,用于标识接收端口号。
- 序列号(Sequence Number):32位,用于标识发送数据的字节流编号。
- 确认号(Acknowledgment Number):32位,用于标识接收到的数据的字节流编号。
- 数据偏移量(Data Offset):4位,用于标识TCP头部的长度(单位为4字节)。
- 保留位(Reserved):6位,保留字段。
- 标志位(Flags):6位,用于标识TCP的状态,主要包括URG、ACK、PSH、RST、SYN、FIN六种。
- 窗口大小(Window Size):16位,用于标识接收方的窗口大小。
- 校验和(Checksum):16位,用于校验TCP头部和数据的完整性。
- 紧急指针(Urgent Pointer):16位,用于标识紧急数据的偏移量。
- 选项(Options):可变长度,用于扩展TCP头部功能,例如选择确认(Selective Acknowledgement)和时间戳(Timestamp)等。
相关问题
用C语言写个IPv6 TCP header checksum计算代码
我可以回答这个问题。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <netinet/ip6.h>
#include <netinet/tcp.h>
unsigned short calculate_tcp_checksum(struct ip6_hdr *ip6hdr, struct tcphdr *tcphdr) {
unsigned short *buf;
unsigned int length = sizeof(struct tcphdr);
unsigned int sum = 0;
buf = (unsigned short *)tcphdr;
while (length > 1) {
sum += *buf++;
length -= 2;
}
if (length == 1) {
sum += *((unsigned char *)buf);
}
buf = (unsigned short *)&ip6hdr->ip6_src;
length = sizeof(struct in6_addr) * 2;
while (length > 1) {
sum += *buf++;
length -= 2;
}
if (length == 1) {
sum += *((unsigned char *)buf);
}
buf = (unsigned short *)&ip6hdr->ip6_dst;
length = sizeof(struct in6_addr) * 2;
while (length > 1) {
sum += *buf++;
length -= 2;
}
if (length == 1) {
sum += *((unsigned char *)buf);
}
sum += htons(IPPROTO_TCP + ntohs(tcphdr->th_len));
length = (unsigned int)ntohs(tcphdr->th_sum);
sum = ~((sum & 0xffff) + (sum >> 16));
sum += length;
sum = (sum >> 16) + (sum & 0xffff);
sum += (sum >> 16);
return (unsigned short)(~sum);
}
int main() {
struct ip6_hdr ip6hdr;
struct tcphdr tcphdr;
memset(&ip6hdr, 0, sizeof(ip6hdr));
memset(&tcphdr, 0, sizeof(tcphdr));
// 填充 IPv6 头部和 TCP 头部
unsigned short checksum = calculate_tcp_checksum(&ip6hdr, &tcphdr);
printf("TCP checksum: 0x%x\n", checksum);
return 0;
}
```
这个代码使用了 `netinet/ip6.h` 和 `netinet/tcp.h` 头文件中定义的结构体,计算 TCP 头部的校验和。
写一个tcp自定义传输协议的结构体,要求有源地址和目标地址,以及配套的相关函数
好的,下面是一个简单的 tcp 自定义传输协议结构体,满足您的要求:
```
struct MyTCPHeader {
uint32_t source_address;
uint32_t destination_address;
uint16_t data_length;
uint16_t checksum;
// 其它需要自定义的字段
// ...
};
// 发送数据时调用此函数填充自定义头部
void fill_my_tcp_header(MyTCPHeader* header, uint32_t src_addr, uint32_t dst_addr, uint16_t datalen) {
header->source_address = htonl(src_addr);
header->destination_address = htonl(dst_addr);
header->data_length = htons(datalen);
header->checksum = 0; // 此处可以计算校验和
}
// 接收数据时调用此函数解析自定义头部
bool parse_my_tcp_header(const uint8_t* buf, size_t buflen, MyTCPHeader* header) {
if (buflen < sizeof(MyTCPHeader)) return false;
memcpy(header, buf, sizeof(MyTCPHeader));
header->source_address = ntohl(header->source_address);
header->destination_address = ntohl(header->destination_address);
header->data_length = ntohs(header->data_length);
// 此处可以验证校验和是否正确
// ...
return true;
}
```
这个结构体包含了源地址和目标地址,同时提供了填充和解析头部信息的函数。当然,这只是一个简单的示例代码,实际应用中还需要更加完善的错误处理和校验功能。
希望这个回答可以帮助到您!
阅读全文
相关推荐
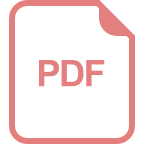
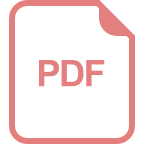
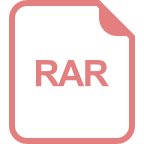
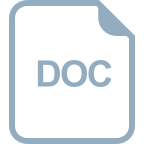
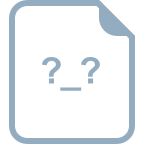
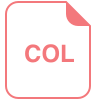
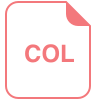
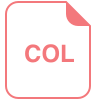
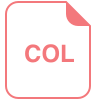






