c语言双向链表实现图书管理系统
时间: 2023-07-13 16:41:30 浏览: 76
好的,下面是一个简单的C语言双向链表实现图书管理系统的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Book {
char name[50];
char author[50];
int year;
struct Book *prev;
struct Book *next;
} Book;
Book *head = NULL;
Book *tail = NULL;
void addBook(char name[], char author[], int year) {
Book *newBook = (Book*) malloc(sizeof(Book));
strcpy(newBook->name, name);
strcpy(newBook->author, author);
newBook->year = year;
newBook->prev = tail;
newBook->next = NULL;
if (head == NULL) {
head = newBook;
} else {
tail->next = newBook;
}
tail = newBook;
}
void removeBook(char name[]) {
Book *current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
if (current == head) {
head = current->next;
} else {
current->prev->next = current->next;
}
if (current == tail) {
tail = current->prev;
} else {
current->next->prev = current->prev;
}
free(current);
return;
}
current = current->next;
}
printf("Book not found.\n");
}
void displayBooks() {
Book *current = head;
while (current != NULL) {
printf("Name: %s\n", current->name);
printf("Author: %s\n", current->author);
printf("Year: %d\n", current->year);
printf("\n");
current = current->next;
}
}
int main() {
addBook("The Great Gatsby", "F. Scott Fitzgerald", 1925);
addBook("To Kill a Mockingbird", "Harper Lee", 1960);
addBook("1984", "George Orwell", 1949);
displayBooks();
removeBook("To Kill a Mockingbird");
displayBooks();
return 0;
}
```
这段代码实现了三个主要的操作:添加书籍、删除书籍和显示所有书籍。每本书都被表示为一个 `Book` 结构体,其中包含书名、作者、出版年份和指向前一本书和后一本书的指针。`addBook` 函数将新书添加到链表的末尾,`removeBook` 函数从链表中删除指定的书籍,`displayBooks` 函数遍历整个链表并打印每本书的信息。
你可以根据需要修改这段代码以适应自己的需求。
相关推荐
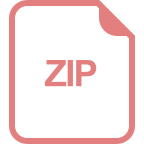
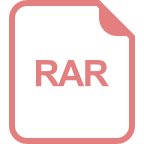














