编程: 创建一个数据库,用于存放下表中学生的姓名、学号、各科目成绩;并打印出每个学生的 姓名、学号、各科目成绩。 a. 使用typedef定义结构体 b. 使用AllocatePage()/AllocatePool()和FreePage()/FreePool()
时间: 2024-12-23 13:16:42 浏览: 10
在编程中,创建一个数据库来存储学生信息通常涉及使用数据结构和内存管理。这里我会分别解释如何使用`typedef`定义结构体以及涉及内存分配的函数。
**a. 使用typedef定义结构体**
首先,我们可以使用`typedef`定义一个结构体,它将包含学生的姓名、学号和各科目的成绩:
```c
// 定义typedef结构体
typedef struct {
char name[50]; // 姓名
int student_id; // 学号
float scores[5]; // 各科目成绩(假设最多5门)
} Student;
Student* create_student(char* name, int student_id, float scores[]);
```
这里我们定义了一个名为`Student`的结构体,并指定了其字段。然后创建一个接受姓名、学号和成绩的函数作为返回值类型,实际创建结构体实例。
**b. 使用内存分配函数**
如果你需要动态分配内存以存储多个学生,可以使用操作系统提供的内存分配函数如`AllocatePage()`和`FreePage()`(这通常是系统级别的功能,在Windows上常见)。但在标准C库中,一般我们会使用`malloc()`和`free()`来进行这样的操作:
```c
Student* create_student(char* name, int student_id, float scores[]) {
Student* new_student = (Student*) malloc(sizeof(Student)); // 动态分配内存
if (new_student != NULL) {
strcpy(new_student->name, name);
new_student->student_id = student_id;
for (int i = 0; i < 5; i++) {
new_student->scores[i] = scores[i];
}
} else {
printf("Memory allocation failed.\n");
return NULL;
}
return new_student;
}
void print_student_info(Student* student) {
if (student == NULL) {
printf("Invalid student pointer.\n");
return;
}
printf("Name: %s\n", student->name);
printf("Student ID: %d\n", student->student_id);
printf("Scores: ");
for (int i = 0; i < 5; i++) {
printf("%f ", student->scores[i]);
}
printf("\n");
}
void free_student_memory(Student* student) {
free(student); // 释放之前分配的内存
}
```
在这个例子中,`create_student()`函数负责分配内存,`print_student_info()`用于打印学生信息,而`free_student_memory()`则负责释放内存。
阅读全文
相关推荐
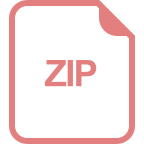
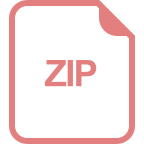
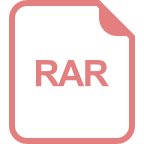
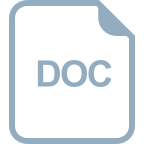
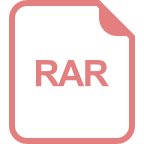
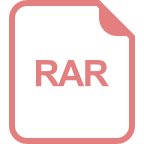
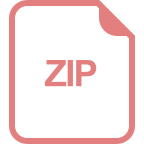
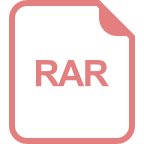
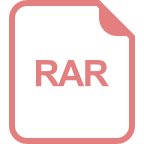
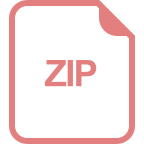
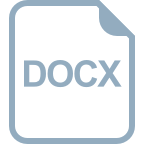
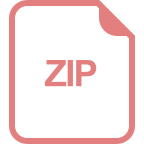
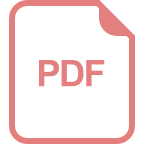
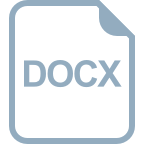
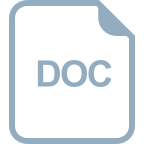
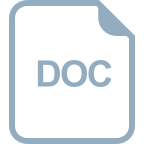