通过文件MultipartFile下载文件到本地
时间: 2023-11-29 22:46:16 浏览: 85
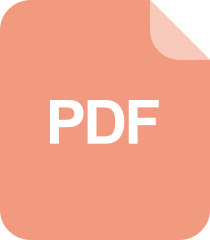
SpringMVC 上传文件 MultipartFile 转为 File的方法
以下是通过MultipartFile下载文件到本地的Python代码示例:
```python
import requests
url = 'http://example.com/file.pdf' # 文件下载链接
response = requests.get(url)
with open('file.pdf', 'wb') as f:
f.write(response.content)
```
其中,`url`是文件下载链接,`response`是通过`requests`库发送GET请求后得到的响应对象,`response.content`是响应内容的二进制形式。我们可以将响应内容写入到本地文件中,从而实现文件下载。
阅读全文
相关推荐
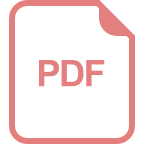
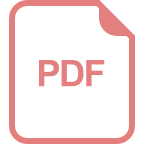















