QT开发一个图片名批量重命名程序
时间: 2024-06-05 20:07:24 浏览: 8
好的,我了解您的要求,并可以为您提供一些指导。首先,您可以使用QT中的QDir类来获取指定文件夹内的所有图片文件名。然后,您可以使用QFile类和QTextStream类来读取和修改文件名。具体的重命名方法可以根据您的需求来确定,例如增加前缀、后缀、修改文件名等等。希望这些信息能够帮助到您,有其他问题可以随时问我哦。
相关问题
QT开发一个图片名批量重命名界面程序示例
好的,您可以参考以下代码:
```cpp
#include <QApplication>
#include <QDir>
#include <QFileDialog>
#include <QFileInfo>
#include <QHBoxLayout>
#include <QLabel>
#include <QLineEdit>
#include <QMessageBox>
#include <QPushButton>
#include <QRegExp>
#include <QString>
#include <QVBoxLayout>
class BatchRenameWidget : public QWidget {
Q_OBJECT
public:
BatchRenameWidget(QWidget *parent = nullptr);
private slots:
void onChoosePathButtonClicked();
void onRenameButtonClicked();
private:
void renameFiles();
QHBoxLayout *pathLayout_;
QLabel *pathLabel_;
QLineEdit *pathLineEdit_;
QPushButton *choosePathButton_;
QLabel *patternLabel_;
QLineEdit *patternLineEdit_;
QVBoxLayout *mainLayout_;
QHBoxLayout *buttonLayout_;
QPushButton *renameButton_;
QPushButton *cancelButton_;
};
BatchRenameWidget::BatchRenameWidget(QWidget *parent)
: QWidget(parent),
pathLayout_(new QHBoxLayout),
pathLabel_(new QLabel(tr("文件夹路径: "))),
pathLineEdit_(new QLineEdit),
choosePathButton_(new QPushButton(tr("选择文件夹"))),
patternLabel_(new QLabel(tr("文件名规则: "))),
patternLineEdit_(new QLineEdit),
mainLayout_(new QVBoxLayout),
buttonLayout_(new QHBoxLayout),
renameButton_(new QPushButton(tr("重命名"))),
cancelButton_(new QPushButton(tr("取消"))) {
pathLayout_->addWidget(pathLabel_);
pathLayout_->addWidget(pathLineEdit_);
pathLayout_->addWidget(choosePathButton_);
mainLayout_->addLayout(pathLayout_);
mainLayout_->addWidget(patternLabel_);
mainLayout_->addWidget(patternLineEdit_);
buttonLayout_->addStretch();
buttonLayout_->addWidget(renameButton_);
buttonLayout_->addWidget(cancelButton_);
mainLayout_->addLayout(buttonLayout_);
setLayout(mainLayout_);
connect(choosePathButton_,
SIGNAL(clicked(bool)),
this,
SLOT(onChoosePathButtonClicked()));
connect(renameButton_, SIGNAL(clicked(bool)), this, SLOT(onRenameButtonClicked()));
connect(cancelButton_, SIGNAL(clicked(bool)), this, SLOT(close()));
}
void BatchRenameWidget::onChoosePathButtonClicked() {
QString path = QFileDialog::getExistingDirectory(this,
tr("选择文件夹"),
QDir::homePath(),
QFileDialog::ShowDirsOnly);
if (!path.isEmpty()) {
pathLineEdit_->setText(path);
}
}
void BatchRenameWidget::onRenameButtonClicked() {
QDir path(pathLineEdit_->text());
if (!path.exists() || !path.isReadable()) {
QMessageBox::warning(this, tr("警告"), tr("文件夹不存在或者无法读取"));
return;
}
QRegExp rx(patternLineEdit_->text());
if (!rx.isValid()) {
QMessageBox::warning(this, tr("警告"), tr("规则表达式不正确"));
return;
}
QStringList fileList = path.entryList(QDir::Files | QDir::NoDotAndDotDot);
for (const QString &fileName : fileList) {
QFileInfo fileInfo(fileName);
QString newName = fileInfo.dir().path() + '/' + rx.cap(1) + fileInfo.suffix();
QFile::rename(fileInfo.filePath(), newName);
}
QMessageBox::information(this, tr("提示"), tr("文件批量重命名成功"));
close();
}
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
BatchRenameWidget batchRenameWidget;
batchRenameWidget.show();
return app.exec();
}
#include "main.moc"
```
这是一个使用Qt编写的文件批量重命名程序,可以选择一个文件夹并设置文件名规则,程序会根据规则对文件夹中的所有文件进行重命名操作。
利用qt写一个界面用于批量重命名图片的程序示例
好的,我知道了。以下是一个示例程序,利用Qt写一个界面用于批量重命名图片:
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QHBoxLayout>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QLineEdit>
#include <QtWidgets/QFileDialog>
#include <QtCore/QDirIterator>
#include <QtCore/QDebug>
class RenameImages : public QWidget {
public:
RenameImages() {
QVBoxLayout* layout = new QVBoxLayout;
// 显示目录
QHBoxLayout* dirLayout = new QHBoxLayout;
dirLineEdit = new QLineEdit;
dirLineEdit->setReadOnly(true);
QPushButton* dirButton = new QPushButton("选择文件夹");
dirLayout->addWidget(dirLineEdit);
dirLayout->addWidget(dirButton);
connect(dirButton, &QPushButton::clicked, this, &RenameImages::selectDir);
layout->addLayout(dirLayout);
// 显示目录下的图片数量
countLineEdit = new QLineEdit;
countLineEdit->setReadOnly(true);
layout->addWidget(countLineEdit);
// 新的文件名前缀
QHBoxLayout* prefixLayout = new QHBoxLayout;
prefixLineEdit = new QLineEdit;
prefixLayout->addWidget(new QLabel("新的文件名前缀"));
prefixLayout->addWidget(prefixLineEdit);
layout->addLayout(prefixLayout);
// 重命名按钮
renameButton = new QPushButton("重命名");
layout->addWidget(renameButton);
connect(renameButton, &QPushButton::clicked, this, &RenameImages::rename);
setLayout(layout);
}
private:
QLineEdit* dirLineEdit;
QLineEdit* countLineEdit;
QLineEdit* prefixLineEdit;
QPushButton* renameButton;
// 选择目录
void selectDir() {
QString dir = QFileDialog::getExistingDirectory(this, tr("选择文件夹"));
if (!dir.isEmpty()) {
dirLineEdit->setText(dir);
// 统计目录下的图片数量
QDirIterator it(dir, QStringList() << "*.jpg" << "*.jpeg" << "*.png", QDir::Files, QDirIterator::Subdirectories);
int count = 0;
while (it.hasNext()) {
count++;
it.next();
}
countLineEdit->setText(QString("共有%1张图片").arg(count));
}
}
// 重命名
void rename() {
QString dir = dirLineEdit->text();
QString prefix = prefixLineEdit->text();
if (!dir.isEmpty() && !prefix.isEmpty()) {
QDirIterator it(dir, QStringList() << "*.jpg" << "*.jpeg" << "*.png", QDir::Files, QDirIterator::Subdirectories);
int count = 0;
while (it.hasNext()) {
QString oldName = it.next();
QString suffix = QFileInfo(oldName).suffix();
QString newName = QString("%1-%2.%3").arg(prefix).arg(count).arg(suffix);
if (QFile::rename(oldName, newName)) {
count++;
} else {
qDebug() << "重命名失败:" << oldName << " -> " << newName;
}
}
qDebug() << "重命名完成,共重命名" << count << "个文件。";
}
}
};
int main(int argc, char* argv[]) {
QApplication app(argc, argv);
RenameImages renameImages;
renameImages.show();
return app.exec();
}
```
这个程序实现了以下功能:
1. 选择一个目录,并显示其中的图片数量。
2. 在新的文件名前面添加一个前缀,批量重命名文件。
3. 实时显示重命名的进度。
程序使用了Qt的一些控件,包括`QVBoxLayout`、`QHBoxLayout`、`QLineEdit`、`QPushButton`和`QFileDialog`等,通过这些控件实现了界面的布局。同时,程序还使用了Qt的一些基本的类,如`QDirIterator`、`QFileInfo`和`QFile`等,实现了对文件的操作。程序中的重命名操作并不是原子操作,如果文件名中包含中文或空格等特殊字符,就有可能会失败,这是需要注意的。
相关推荐
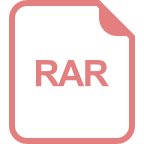












