jupyter 风格迁移
时间: 2023-06-10 10:08:08 浏览: 115
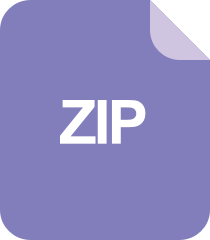
图片风格迁移系统.zip

Jupyter 风格迁移是一种将一张图片的风格应用到另一张图片上的技术。这个技术基于深度学习模型,可以将一张图片的风格转移到另一张图片上,从而创造出新的风格独特的图片。在 Jupyter 中,可以使用 Python 的深度学习框架如 TensorFlow 或 PyTorch 来实现风格迁移。
以下是一个简单的 Jupyter 风格迁移的代码示例:
```python
import tensorflow as tf
import numpy as np
import PIL.Image
import matplotlib.pyplot as plt
# 加载图片
def load_image(img_path, max_size=512):
img = PIL.Image.open(img_path)
if max(img.size) > max_size:
size = max_size
else:
size = max(img.size)
img = img.resize((size, int(size * (img.size[1] / img.size[0]))))
img = np.array(img)
return img
# 显示图片
def show_image(image):
plt.imshow(image.astype('uint8'))
plt.show()
# 加载模型
model = tf.keras.applications.VGG19(include_top=False, weights='imagenet')
# 提取特征
def get_feature_representations(model, content_path, style_path):
content_image = load_image(content_path)
style_image = load_image(style_path)
content_image = tf.keras.applications.vgg19.preprocess_input(content_image)
style_image = tf.keras.applications.vgg19.preprocess_input(style_image)
content_features = model(content_image)
style_features = model(style_image)
return content_features, style_features
# 计算 gram 矩阵
def gram_matrix(input_tensor):
result = tf.linalg.einsum('bijc,bijd->bcd', input_tensor, input_tensor)
shape = tf.shape(input_tensor)
num_locations = tf.cast(shape[1] * shape[2], tf.float32)
return result / (num_locations)
# 计算风格损失
def calculate_style_loss(style_features, generated_features):
style_grams = [gram_matrix(style_feature) for style_feature in style_features]
generated_grams = [gram_matrix(generated_feature) for generated_feature in generated_features]
style_loss = tf.add_n([tf.reduce_mean((style_gram - generated_gram) ** 2) for style_gram, generated_gram in zip(style_grams, generated_grams)])
return style_loss / len(style_features)
# 计算内容损失
def calculate_content_loss(content_features, generated_features):
content_loss = tf.reduce_mean((content_features - generated_features) ** 2)
return content_loss
# 计算总损失
def calculate_total_loss(model, loss_weights, generated_image, content_features, style_features):
content_weight, style_weight = loss_weights
model_outputs = model(generated_image)
content_output = model_outputs[3]
style_outputs = model_outputs[:-1]
content_loss = calculate_content_loss(content_features, content_output)
style_loss = calculate_style_loss(style_features, style_outputs)
total_loss = content_weight * content_loss + style_weight * style_loss
return total_loss
# 计算梯度
def calculate_gradients(model, loss_weights, generated_image, content_features, style_features):
with tf.GradientTape() as tape:
total_loss = calculate_total_loss(model, loss_weights, generated_image, content_features, style_features)
gradients = tape.gradient(total_loss, generated_image)
return gradients
# 迭代更新图片
def run_style_transfer(content_path, style_path, epochs=10, steps_per_epoch=100, content_weight=1e3, style_weight=1e-2):
content_features, style_features = get_feature_representations(model, content_path, style_path)
loss_weights = (content_weight, style_weight)
generated_image = tf.Variable(load_image(content_path))
opt = tf.optimizers.Adam(learning_rate=5, beta_1=0.99, epsilon=1e-1)
for epoch in range(epochs):
for step in range(steps_per_epoch):
gradients = calculate_gradients(model, loss_weights, generated_image, content_features, style_features)
opt.apply_gradients([(gradients, generated_image)])
generated_image.assign(tf.clip_by_value(generated_image, 0, 255))
show_image(generated_image.numpy())
return generated_image
# 运行风格迁移
content_path = 'path/to/content/image'
style_path = 'path/to/style/image'
generated_image = run_style_transfer(content_path, style_path, epochs=10, steps_per_epoch=100, content_weight=1e3, style_weight=1e-2)
```
这段代码会将 `content_path` 中的图片的内容与 `style_path` 中的图片的风格进行结合,并输出一张新的图片。可以通过调整 `epochs`、`steps_per_epoch`、`content_weight` 和 `style_weight` 等参数来调整生成图片的效果。
阅读全文
相关推荐
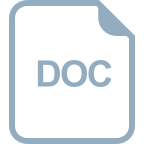
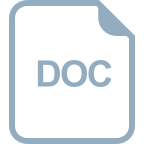

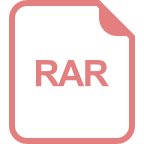
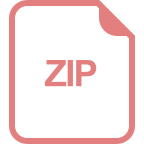
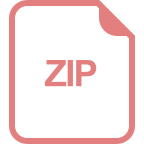
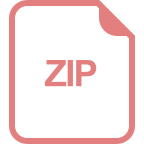
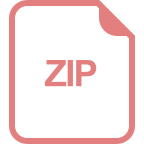
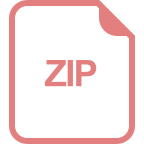
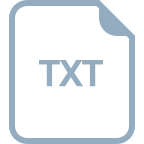
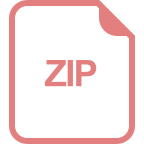
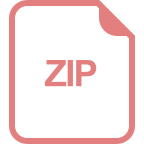
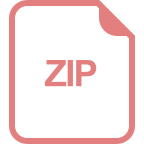
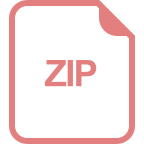
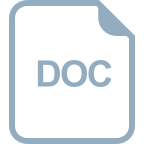
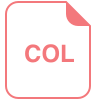
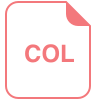
