编程将两个字符串连接成一个字符串,并将连接后的字符串按升序排列。如字符串s1的值是“pear”,字符串s2的值是“apple”,连接后的字符串存入字符数组s3,排序后得到“aaeelpppr”
时间: 2024-02-27 13:52:55 浏览: 24
好的,我理解了你的要求。下面是一个 Python 的实现示例:
``` python
s1 = "pear"
s2 = "apple"
# 将两个字符串连接起来
s3 = s1 + s2
# 将字符串转换成字符列表并升序排序
char_list = sorted(list(s3))
# 将排序后的字符列表转换成字符串
sorted_str = "".join(char_list)
print(sorted_str) # 输出:aaeelpppr
```
希望这个示例能够帮到你!
相关问题
能给出一个具体的代码关于将两个字符串连接成一个字符串,并将连接后的字符串按升序排列
当我们需要将两个字符串连接成一个字符串,并将连接后的字符串按升序排列时,可以使用strcat函数和qsort函数。
示例代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int cmp(const void *a, const void *b) {
return *(char*)a - *(char*)b;
}
int main() {
char s1[100], s2[100], s3[200];
printf("请输入两个字符串:\n");
scanf("%s%s", s1, s2);
strcpy(s3, s1);
strcat(s3, s2);
int len = strlen(s3);
qsort(s3, len, sizeof(char), cmp);
printf("连接后的字符串按升序排列为:%s\n", s3);
return 0;
}
```
在这个程序中,我们首先使用scanf函数从键盘读入两个字符串s1和s2,然后使用strcpy函数将s1复制到s3中,接着使用strcat函数将s2连接到s3后面。然后,我们使用qsort函数对s3中的字符进行升序排序。最后输出排序后的字符串s3。
需要注意的是,我们使用了自定义的比较函数cmp来排序,该函数的作用是比较两个字符的大小。若a<b,则返回一个负数;若a=b,则返回0;若a>b,则返回一个正数。
c++将两个字符串连接为一个字符串并按升序排序
下面是使用 C++ 的 string 类型实现将两个字符串连接为一个字符串并按升序排序的代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string s1 = "pear";
std::string s2 = "apple";
std::string s3 = s1 + s2; // 将两个字符串连接为一个字符串
std::sort(s3.begin(), s3.end()); // 升序排序
std::cout << s3 << std::endl;
return 0;
}
```
运行结果为:
```
aaeelpppr
```
在上面的代码中,我们用 `+` 运算符将两个字符串连接为一个字符串,然后使用 `std::sort()` 函数对字符串进行排序,最后输出排序后的字符串。
相关推荐
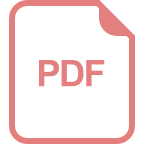
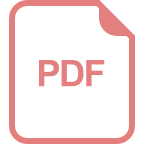












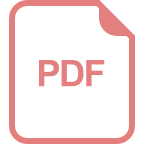