def gaussmf(self, x, c, sigma): return np.exp(-np.power(x - c, 2.) / (2 * np.power(sigma, 2.)))优化该代码
时间: 2023-12-08 10:06:02 浏览: 73
这段代码可以进行如下优化:
1. 使用numpy的内置函数来代替手写的计算:使用`np.exp`函数代替手写的指数计算操作,使用`np.power`函数代替手写的幂运算操作,可以加速代码运行。
2. 使用向量化操作:将一维数组作为输入,可以使用numpy的向量化操作,避免使用循环等低效的操作。
修改后的代码如下:
```python
import numpy as np
class GauMF:
def gaussmf(self, x, c, sigma):
return np.exp(-((x - c) ** 2) / (2 * sigma ** 2))
```
这样修改后的代码可以更快地运行,并且更易于理解和维护。
相关问题
def gaussmf(self, x, c, sigma): return np.exp(-np.power(x - c, 2.) / (2 * np.power(sigma, 2.)))出现perands could not be broadcast together with shapes (32,3) (0,) 修改
根据你提供的代码,我发现在gaussmf函数中,如果x的shape是(32, 3),则会出现perands could not be broadcast together with shapes (32,3) (0,)的错误。这是因为在计算高斯函数时,对于每个输入x,都需要对c和sigma进行高斯函数计算,而c和sigma的shape都是(0,),无法与x进行broadcast。
一种可能的修正方法是,在调用gaussmf函数时,将sigma沿着第0维复制32次,使其与x和c的shape相同:
```
def predict(self, X):
mf_out = np.zeros((len(X), self.n, self.m))
for i in range(self.n):
sigma = np.tile(self.params['mf_params'][self.m:], (len(X), 1))
mf_out[:, i, :] = self.gaussmf(X[:, i].reshape(-1, 1), self.params['mf_params'][:self.m], sigma)
```
这样,就可以避免perands could not be broadcast together with shapes的错误。
修正该代码 def gaussmf(self, x, c, sigma): return np.exp(-np.power(x - c, 2.) / (2 * np.power(sigma, 2.)))
您提供的代码是高斯隶属度函数的实现,看起来没有错误。不过,如果您需要对其进行修正,可以根据具体要求进行修改,例如:
```python
def gaussmf(self, x, c, sigma):
"""
高斯隶属度函数
:param x: 输入变量
:param c: 高斯函数的中心
:param sigma: 高斯函数的标准差
:return: 高斯函数的输出
"""
return np.exp(-0.5 * np.square((x - c) / sigma))
```
这里对原有代码进行了一些微调,将平方操作使用了`np.square()`函数代替,同时将高斯函数的公式进行了简化,以更加清晰地表达其含义。
阅读全文
相关推荐
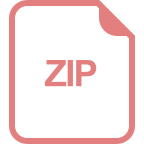
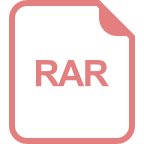
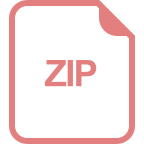
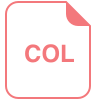
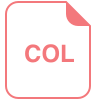
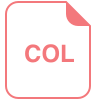
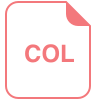


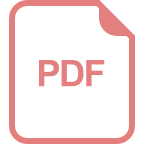
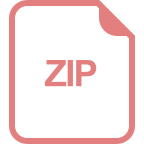
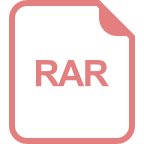
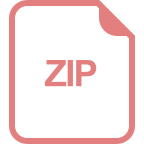
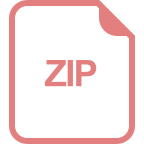
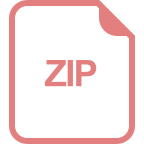
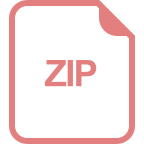