java数据结构链表存储mysql的food表怎么操作
时间: 2024-02-06 07:03:27 浏览: 59
首先,您需要定义一个类来表示food表中的每个行。该类应该包含与food表中的列相对应的实例变量以及它们的getter和setter方法。例如:
```
public class Food {
private int id;
private String name;
private double price;
// Constructor
public Food(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
// Getter and Setter methods
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
接下来,您需要创建一个链表来存储food表中的所有行。您可以使用Java的LinkedList类来实现这个链表。例如:
```
LinkedList<Food> foodList = new LinkedList<Food>();
```
现在,您可以使用Java的JDBC API来连接MySQL数据库并执行SQL查询。以下是一个示例代码块,用于将food表中的所有行添加到LinkedList中:
```
try {
// Load the MySQL JDBC driver
Class.forName("com.mysql.jdbc.Driver");
// Create a connection to the database
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "myuser", "mypassword");
// Create a statement to execute the SQL query
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM food");
// Add each row to the foodList
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
double price = resultSet.getDouble("price");
Food food = new Food(id, name, price);
foodList.add(food);
}
// Close the connection
connection.close();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
```
现在,您可以对foodList执行各种操作,例如遍历列表并打印每个食品的名称和价格:
```
for (Food food : foodList) {
System.out.println(food.getName() + ": " + food.getPrice());
}
```
阅读全文
相关推荐
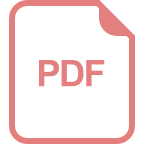
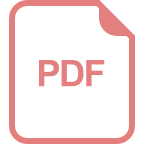
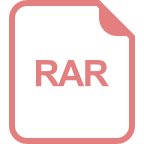
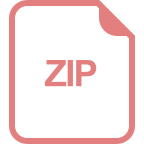
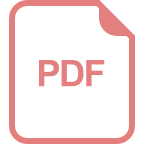
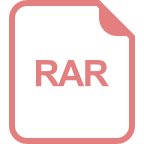
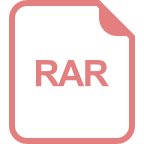
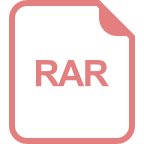
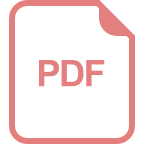
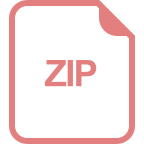
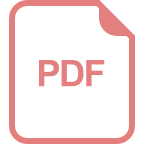
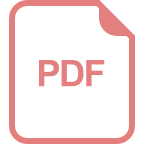