c++拷贝超大文件夹下所有文件到另一文件夹下
时间: 2023-08-04 13:03:59 浏览: 192
在 C++ 中,你可以使用 `boost::filesystem` 库来实现拷贝超大文件夹下所有文件到另一文件夹下的功能。具体代码如下:
```c++
#include <iostream>
#include <boost/filesystem.hpp>
namespace fs = boost::filesystem;
void copy_folder(const fs::path& source, const fs::path& destination)
{
if (!fs::exists(destination))
{
fs::create_directories(destination);
}
for (fs::directory_iterator file(source); file != fs::directory_iterator(); ++file)
{
const fs::path current(file->path());
const fs::path destination_file(destination / current.filename());
if (fs::is_directory(current))
{
copy_folder(current, destination_file);
}
else
{
fs::copy_file(current, destination_file, fs::copy_option::overwrite_if_exists);
}
}
}
int main()
{
const fs::path source("/path/to/source/folder");
const fs::path destination("/path/to/destination/folder");
copy_folder(source, destination);
return 0;
}
```
其中,`copy_folder` 函数使用递归方式遍历源文件夹中的所有文件和子文件夹,并将它们拷贝到目标文件夹中。如果目标文件夹不存在,则先创建目标文件夹。
请将 `/path/to/source/folder` 和 `/path/to/destination/folder` 替换成实际的源文件夹和目标文件夹路径。注意,该代码需要使用 `boost::filesystem` 库,需要在编译时链接该库。
阅读全文
相关推荐
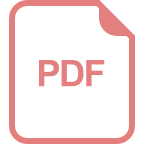






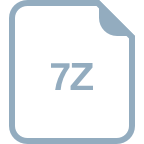
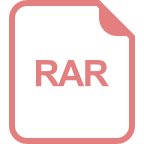
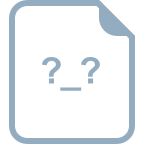
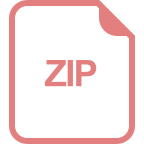
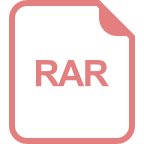
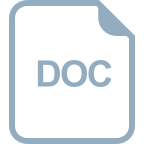
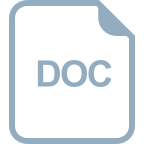

