设计一个学生信息管理系统,实现对学生信息的以下操作: 1、以线性表的顺序存储结构创建学生信息顺序表。 2、以线性表的链式存储结构(单链表)创建学生信息单链表。 3、在这两种存储结构上实现学生数据的插入、删除和按学号查找的算法 用c++
时间: 2024-10-16 07:05:29 浏览: 79
设计一个学生信息管理系统,我们可以使用C++来实现。这里我会给出基本的概念和部分代码示例,包括如何使用顺序表(数组)和链表来存储和操作学生信息。
**一、顺序表实现**
```cpp
#include <iostream>
using namespace std;
// 定义学生结构体
struct Student {
int id;
string name;
// ...其他属性...
};
class SeqStudentInfoManager {
private:
Student* students; // 学生信息数组
int size, capacity;
public:
SeqStudentInfoManager(int cap) {
capacity = cap;
students = new Student[capacity];
size = 0;
}
// 插入方法
void insert(Student s) {
if (size == capacity) resize(capacity * 2);
students[size++] = s;
}
// 删除方法
bool removeByID(int id) {
for (int i = 0; i < size; ++i) {
if (students[i].id == id) {
memmove(students + i, students + i + 1, sizeof(Student) * (size - i - 1));
size--;
return true;
}
}
return false;
}
// 查找方法
Student* findByID(int id) const {
for (int i = 0; i < size; ++i) {
if (students[i].id == id) return &students[i];
}
return nullptr;
}
private:
void resize(int newCap) {
Student* newStudents = new Student[newCap];
for (int i = 0; i < size; ++i)
newStudents[i] = students[i];
delete[] students;
students = newStudents;
capacity = newCap;
}
};
```
**二、链表实现**
```cpp
#include <iostream>
using namespace std;
// 学生节点
struct StudentNode {
int id;
string name;
// ...其他属性...
StudentNode* next;
};
class LinkStudentInfoManager {
private:
StudentNode* head;
public:
LinkStudentInfoManager() { head = nullptr; }
// 插入方法
void insert(Student s) {
StudentNode* newNode = new StudentNode{s.id, s.name}; // 创建新节点
if (!head) {
head = newNode;
} else {
StudentNode* current = head;
while (current->next) current = current->next;
current->next = newNode;
}
}
// 删除方法
bool removeByID(int id) {
StudentNode* prev = nullptr, *current = head;
while (current) {
if (current->id == id) {
if (prev) prev->next = current->next;
else head = current->next;
delete current;
return true;
}
prev = current;
current = current->next;
}
return false;
}
// 查找方法
StudentNode* findByID(int id) {
StudentNode* current = head;
while (current) {
if (current->id == id) return current;
current = current->next;
}
return nullptr;
}
};
```
阅读全文
相关推荐




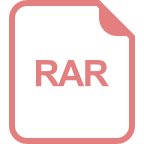









