中望cad的pline命令画圆弧功能用c++代码表示
时间: 2024-03-15 08:47:54 浏览: 20
以下是使用C++代码实现中望CAD的Pline命令画圆弧功能的示例:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义圆弧结构体
struct Arc {
double start_x;
double start_y;
double end_x;
double end_y;
double center_x;
double center_y;
double radius;
double start_angle;
double end_angle;
};
// 计算圆心坐标和半径
Arc calculateArc(double start_x, double start_y, double end_x, double end_y, double radius, int direction) {
Arc arc;
double d = sqrt(pow(end_x - start_x, 2) + pow(end_y - start_y, 2));
double a = asin((end_y - start_y) / d);
double b = acos((start_x - end_x) / (2 * radius));
double c = direction * (a + b);
arc.start_x = start_x;
arc.start_y = start_y;
arc.end_x = end_x;
arc.end_y = end_y;
arc.center_x = start_x + radius * sin(c);
arc.center_y = start_y + radius * cos(c);
arc.radius = radius;
arc.start_angle = atan2(start_y - arc.center_y, start_x - arc.center_x);
arc.end_angle = atan2(end_y - arc.center_y, end_x - arc.center_x);
return arc;
}
int main() {
// 模拟用户输入的起点、终点和半径
double start_x = 0;
double start_y = 0;
double end_x = 5;
double end_y = 5;
double radius = 3;
// 计算圆心坐标和半径
Arc arc = calculateArc(start_x, start_y, end_x, end_y, radius, 1);
// 输出圆心坐标和半径
cout << "Center point: (" << arc.center_x << ", " << arc.center_y << ")" << endl;
cout << "Radius: " << arc.radius << endl;
return 0;
}
```
通过调用calculateArc函数来计算圆心坐标和半径,其中参数包括起点、终点、半径和方向(1表示逆时针,-1表示顺时针),返回值是一个包含圆心坐标和半径等信息的Arc结构体。在主函数中,我们模拟了用户输入的起点、终点和半径,然后调用calculateArc函数来计算圆心坐标和半径,并输出结果。注意,这里只是一个示例,实际应用中还需要考虑更多的因素。
相关推荐
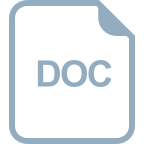
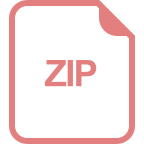
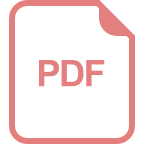














