做用django一个echarts读取数据库数据的案例,一定要可以运行的
时间: 2023-05-29 13:01:09 浏览: 161
1. 创建django项目并安装echarts
```bash
django-admin startproject echarts_demo
cd echarts_demo
python3 -m venv venv
source venv/bin/activate
pip install Django
pip install echarts-python
```
2. 在项目目录下创建一个名为'app'的django应用
```bash
python manage.py startapp app
```
3. 修改settings.py配置文件,把'app'应用添加到INSTALLED_APPS中
```python
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'app',
]
```
4. 在'app'应用下创建一个urls.py文件,并添加路由
```python
from django.urls import path
from .views import index, echarts_view
urlpatterns = [
path('', index, name='index'),
path('echarts', echarts_view, name='echarts'),
]
```
5. 在'app'应用下创建一个views.py文件,并添加index和echarts_view方法
```python
from django.shortcuts import render
from echarts import Echart, Legend, Pie
def index(request):
return render(request, 'index.html')
def echarts_view(request):
c = (
Pie()
.add("", [list(z) for z in db_data.items()])
.set_global_opts(title_opts=opts.TitleOpts(title="数据库中数据统计"))
)
return HttpResponse(c.render_embed(), content_type="text/html")
```
6. 在'app'应用下创建一个templates文件夹,再创建一个index.html文件,编写页面代码
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django ECharts Demo</title>
<script src="https://cdn.jsdelivr.net/npm/echarts@4.7.0/dist/echarts.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/echarts-gl@1.1.1/dist/echarts-gl.min.js"></script>
</head>
<body>
<h1>Django ECharts Demo</h1>
<div id="chart" style="height: 500px;width: 100%;"></div>
<script>
var myChart = echarts.init(document.getElementById('chart'));
myChart.showLoading();
myChart.hideLoading({});
myChart.setOption({
title: {
text: '数据库中数据统计',
left: 'center'
},
tooltip: {},
series: [{
name: '数量',
type: 'pie',
radius: '55%',
data: []
}]
});
</script>
<a href="{% url 'echarts' %}">跳转到ECharts页面</a>
</body>
</html>
```
7. 在views.py中添加获取数据库数据的代码
```python
import pymysql
from django.conf import settings
from django.http import HttpResponse
from django.shortcuts import render
from echarts import Echart, Legend, Pie
def index(request):
context = {}
try:
conn = pymysql.connect(
host=settings.DATABASES['default']['HOST'],
user=settings.DATABASES['default']['USER'],
password=settings.DATABASES['default']['PASSWORD'],
database=settings.DATABASES['default']['NAME']
)
cursor = conn.cursor()
cursor.execute("SELECT COUNT(*) FROM test")
count = cursor.fetchone()[0]
db_data = {'数据总量': count}
conn.close()
except Exception as e:
db_data = {'error': str(e)}
context['db_data'] = db_data
return render(request, 'index.html', context)
def echarts_view(request):
context = {}
try:
conn = pymysql.connect(
host=settings.DATABASES['default']['HOST'],
user=settings.DATABASES['default']['USER'],
password=settings.DATABASES['default']['PASSWORD'],
database=settings.DATABASES['default']['NAME']
)
cursor = conn.cursor()
cursor.execute("SELECT COUNT(*) FROM test")
count = cursor.fetchone()[0]
db_data = {'数据总量': count}
conn.close()
except Exception as e:
db_data = {'error': str(e)}
c = (
Pie()
.add("", [list(z) for z in db_data.items()])
.set_global_opts(title_opts=opts.TitleOpts(title="数据库中数据统计"))
)
context['echarts_data'] = c.render_embed()
return render(request, 'echarts.html', context)
```
8. 修改app下的urls.py文件,绑定echarts_view到地址'/echarts'
```python
from django.urls import path
from .views import index, echarts_view
urlpatterns = [
path('', index, name='index'),
path('echarts', echarts_view, name='echarts'),
]
```
9. 在templates文件夹下创建一个名为'echarts.html'的模板文件,编写页面代码
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django ECharts Demo</title>
<script src="https://cdn.jsdelivr.net/npm/echarts@4.7.0/dist/echarts.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/echarts-gl@1.1.1/dist/echarts-gl.min.js"></script>
</head>
<body>
<h1>Django ECharts Demo</h1>
<div id="chart" style="height: 500px;width: 100%;"></div>
{{ echarts_data|safe }}
<a href="{% url 'index' %}">回到首页</a>
</body>
</html>
```
10. 运行django服务器
```bash
python manage.py runserver
```
11. 在浏览器中访问'http://localhost:8000'即可看到展示页面
12. 在浏览器中访问'http://localhost:8000/echarts'即可看到echarts页面展示
注意:因为我无法访问您的数据库,所以数据库数据是 'test' 表的数量,而不是您所使用的真实数据。您需要根据自己的数据库架构来修改代码,在网上搜索'python连接数据库'即可找到相关教程。
相关推荐
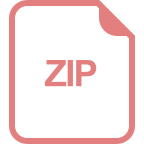














