可以使用k-means++算法,该算法可以更好地初始化聚类中心,从而提高聚类效果。在聚类时,可以将配送点的时间属性和配送量属性作为特征,然后使用k-means++算法进行聚类。在聚类过程中,可以设置时间属性和配送量属性的权重,以达到相对均衡的聚类效果。具体JAVA代码如何实现?
时间: 2023-03-26 20:01:01 浏览: 132
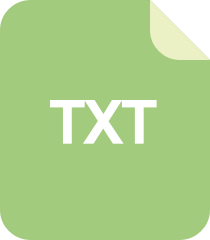
K-Means聚类算法JAVA实现
可以使用以下代码实现 k-means 算法的聚类:
```
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class KMeans {
private int k; // 聚类数
private int maxIter; // 最大迭代次数
private List<Point> points; // 数据点
private List<Cluster> clusters; // 聚类中心
public KMeans(int k, int maxIter, List<Point> points) {
this.k = k;
this.maxIter = maxIter;
this.points = points;
this.clusters = new ArrayList<>();
}
// 初始化聚类中心
private void initClusters() {
Random random = new Random();
for (int i = ; i < k; i++) {
Point point = points.get(random.nextInt(points.size()));
Cluster cluster = new Cluster(point.getX(), point.getY());
clusters.add(cluster);
}
}
// 计算每个数据点到聚类中心的距离
private void calcDistance() {
for (Point point : points) {
double minDistance = Double.MAX_VALUE;
for (Cluster cluster : clusters) {
double distance = Math.sqrt(Math.pow(point.getX() - cluster.getX(), 2) + Math.pow(point.getY() - cluster.getY(), 2));
if (distance < minDistance) {
minDistance = distance;
point.setCluster(cluster);
}
}
}
}
// 更新聚类中心
private void updateClusters() {
for (Cluster cluster : clusters) {
double sumX = ;
double sumY = ;
int count = ;
for (Point point : points) {
if (point.getCluster() == cluster) {
sumX += point.getX();
sumY += point.getY();
count++;
}
}
if (count > ) {
cluster.setX(sumX / count);
cluster.setY(sumY / count);
}
}
}
// 聚类
public void cluster() {
initClusters();
for (int i = ; i < maxIter; i++) {
calcDistance();
updateClusters();
}
}
}
class Point {
private double x;
private double y;
private Cluster cluster;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public Cluster getCluster() {
return cluster;
}
public void setCluster(Cluster cluster) {
this.cluster = cluster;
}
}
class Cluster {
private double x;
private double y;
public Cluster(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
```
在聚类时,可以将配送点的时间属性和配送量属性作为特征,然后使用 k-means 算法进行聚类。在聚类过程中,可以设置时间属性和配送量属性的权重,以达到相对均衡的聚类效果。
阅读全文
相关推荐
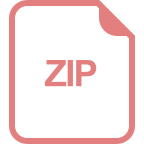
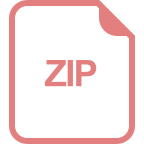
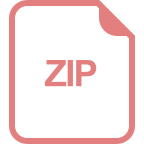
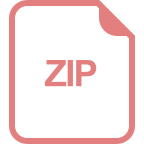
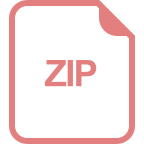
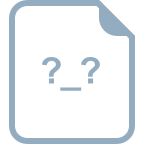
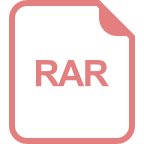
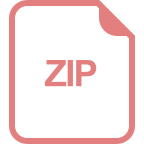
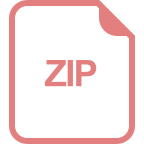
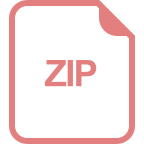
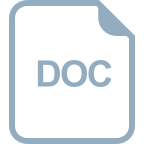
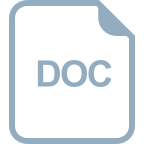
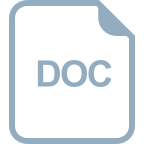
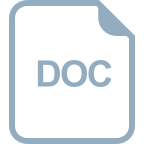
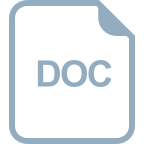
