写一个函数add_tuple(t),参数t是一个元组,取t的第2个到第5个(包含)元素,然后在这些元素的前面增加一个元素'a',形成一个新的元组,返回这个新的元组。
时间: 2024-02-01 20:16:18 浏览: 12
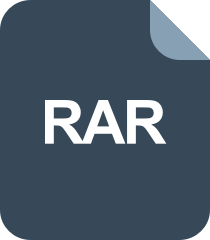
在数组中添加一个元素

可以使用元组切片和元组拼接来实现:
```python
def add_tuple(t):
return ('a',) + t[1:5]
```
这里使用了`('a',)`来创建只包含一个元素的元组,因为括号内只有一个元素时,需要在后面加上逗号,否则会被解释为普通的括号。
阅读全文
相关推荐
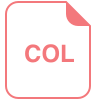
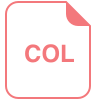

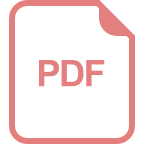
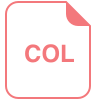
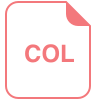
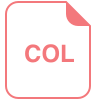
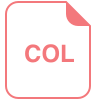







