帮我用python写一个程序用于解决矫正图片亮度不均匀的问题
时间: 2023-12-04 21:02:47 浏览: 34
好的,我可以帮您用Python编写一个程序来解决矫正图片亮度不均匀的问题。您可以使用OpenCV库来实现这个功能,具体步骤如下:
1. 读取输入图像
2. 转换成灰度图像
3. 计算图像的全局亮度均值和标准差
4. 计算每个像素的局部亮度均值和标准差
5. 对每个像素进行亮度矫正
6. 输出处理后的图像
以下是Python代码示例:
import cv2
def uneven_lighting_correction(image):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Calculate global mean and standard deviation of pixel values
global_mean, global_stddev = cv2.meanStdDev(gray)
# Define the size of local region for calculating mean and standard deviation
block_size = 64
# Calculate the size of each block
height, width = gray.shape[:2]
num_blocks_y = height // block_size
num_blocks_x = width // block_size
# Loop over each block
for y in range(num_blocks_y):
for x in range(num_blocks_x):
# Calculate the coordinates of the block
x1 = x * block_size
y1 = y * block_size
x2 = x1 + block_size
y2 = y1 + block_size
# Extract the block from the grayscale image
block = gray[y1:y2, x1:x2]
# Calculate the mean and standard deviation of pixel values for the block
block_mean, block_stddev = cv2.meanStdDev(block)
# Calculate the scaling factor for the block
scale = global_stddev / block_stddev
offset = global_mean - scale * block_mean
# Apply the scaling factor and offset to the block
corrected_block = cv2.convertScaleAbs(block, alpha=scale, beta=offset)
# Replace the block in the grayscale image with the corrected block
gray[y1:y2, x1:x2] = corrected_block
# Convert the grayscale image back to BGR format
corrected = cv2.cvtColor(gray, cv2.COLOR_GRAY2BGR)
return corrected
# Load image
image = cv2.imread('input.jpg')
# Perform uneven lighting correction
corrected_image = uneven_lighting_correction(image)
# Save output image
cv2.imwrite('output.jpg', corrected_image)
请注意,这个程序只能处理亮度不均的问题,如果图片存在其他问题,可能需要进行其他处理。
相关推荐
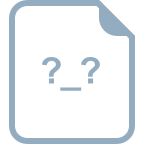
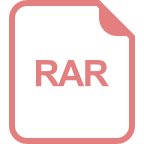














