import matplotlib as mpl import matplotlib.pyplot as plt plt.subplots_adjust(left=None, bottom=None, right=None, top=None, wspace=None, hspace=0.5) t=np.arange(0.0,2.0,0.1) s=np.sin(t*np.pi) plt.subplot(2,2,1) #要生成两行两列,这是第一个图 import numpy as np import matplotlib.pyplot as plt x = np.arange(1,13) y1 = np.array([53673, 57571, 58905, 55239, 49661, 49510, 49163, 57311, 59187, 60074, 57109, 52885]) plt.plot(x, y1) plt.title('近13天登录人数') plt.show() plt.subplot(2,2,2) #两行两列,这是第二个图 import numpy as np import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) x = np.arange(12) y = np.array([70887, 64957, 62508, 66471, 54972, 46245, 64083, 67090, 64991, 88504, 79404, 68253,]) bar_width = 0.3 plt.bar(x, y, tick_label=['1月', '2月', '3月', '4月', '5月', '6月', '7月', "8月", "9月", "10月", '11月', '12月'],width=bar_width) plt.title('近12个月人数') plt.show() plt.subplot(2,2,3)#两行两列,这是第三个图 import matplotlib.pyplot as plt plt.subplots_adjust(top=0.85) plt.style.use('fivethirtyeight') languages = ['steam', 'ubisoft'] popularity = [78,22] plt.pie(popularity) plt.tight_layout() plt.pie(popularity, labels=languages, autopct='%1.1f%%') plt.title('游戏平台登录占比') plt.subplot(2,2,4)#两行两列,这是第四个图 import numpy as np from matplotlib import pyplot as plt ages_x = [1, 2, 3, 4, 5, ] x_indexes = np.arange(len(ages_x)) width = 0.33 dev_y = [64050, 65168, 75588, 75590, 60097] py_dev_y = [57809, 56101, 70605, 63437, 56756] plt.bar(x_indexes, dev_y, width=width, label="2022") plt.bar(x_indexes + width, py_dev_y, width=width, label="2023") plt.xlabel("月份") plt.ylabel("平均在线人数") plt.title("2022和2023一到五月在线人数对比") plt.legend() plt.xticks(ticks = x_indexes, labels = ages_x) plt.show()
时间: 2023-10-09 12:08:17 浏览: 107
你刚才的代码中,第三个子图中的饼图有一些问题。虽然你使用了pie函数绘制了饼图,但是没有正确地添加标签和百分比。正确的代码应该是这样的:
```python
plt.subplot(2,2,3)
plt.subplots_adjust(top=0.85)
plt.style.use('fivethirtyeight')
languages = ['steam', 'ubisoft']
popularity = [78,22]
plt.pie(popularity, labels=languages, autopct='%1.1f%%')
plt.title('游戏平台登录占比')
plt.show()
```
这样做可以让饼图正确地显示游戏平台的登录占比,并显示出每个部分所占的百分比。
相关问题
import matplotlib as mpl import matplotlib.pyplot as plt
这段代码是导入 Matplotlib 库,并将其别名为 mpl,同时导入 pyplot 模块并将其别名为 plt。Matplotlib 是一个用于绘制数据可视化图形的 Python 库,pyplot 模块是 Matplotlib 中的一个子模块,提供了类似于 MATLAB 绘图命令的简单界面,可以进行快速的绘图。
import numpy as np import matplotlib.pyplot as plt from mpl_toolkits.mplot3d
import numpy是一个用于科学计算的Python库,它提供了大量用于数组操作和数学计算的函数和工具。使用import numpy as np将numpy库导入为np别名,以便在代码中更方便地使用numpy的函数和工具。
而import matplotlib.pyplot as plt是导入matplotlib库的一种常用方式,matplotlib是一个用于绘制图表和数据可视化的库,import语句将matplotlib.pyplot模块导入为plt别名,以便在代码中更方便地使用matplotlib库的绘图函数和工具。
最后,from mpl_toolkits.mplot3d导入模块同样是用于matplotlib库的一种常见操作,它用于导入mpl_toolkits.mplot3d模块,这是一个用于绘制三维图表和可视化三维数据的工具模块。
综合起来,这些import语句在Python代码中的作用是将numpy和matplotlib库以及其相关的模块导入到代码中,以便使用它们提供的各种函数和工具进行数组操作、数学计算和绘制图表等操作。这样可以极大地方便数据科学家、工程师和研究人员们对数据的处理和可视化工作。
相关推荐
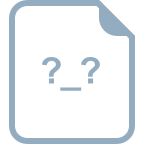
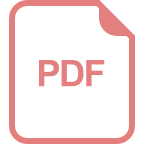
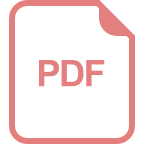












