jupyter爬取网页数据
时间: 2023-10-06 16:12:56 浏览: 190
要在 Jupyter 中爬取网页数据,你需要使用一些 Python 库来实现这个目标。其中最常用的是 requests 和 BeautifulSoup。
1. 首先,需要安装这些库。在 Jupyter 中,可以使用以下命令来安装:
```python
!pip install requests
!pip install beautifulsoup4
```
2. 导入这些库:
```python
import requests
from bs4 import BeautifulSoup
```
3. 使用 requests 库获取网页内容:
```python
url = "https://www.example.com"
response = requests.get(url)
content = response.content
```
4. 使用 BeautifulSoup 库解析网页内容:
```python
soup = BeautifulSoup(content, "html.parser")
```
5. 使用 BeautifulSoup 的方法来获取需要的数据。例如,如果你要获取网页中的标题,可以使用以下代码:
```python
title = soup.title.string
print(title)
```
以上是一个简单的爬取网页数据的示例,你可以根据自己的需求进行修改和扩展。不过需要注意的是,在爬取网页数据时,需要遵守网站的规定和法律法规,避免侵犯他人的权益。
相关问题
jupyter爬取天气数据
使用Jupyter Notebook来爬取天气数据是一个很常见的任务。你可以使用Python的requests库来发送HTTP请求获取网页内容,然后使用BeautifulSoup库来解析HTML,从而提取所需的天气数据。
首先,你需要安装所需的库。在Jupyter Notebook中,你可以使用以下命令来安装它们:
```python
!pip install requests
!pip install beautifulsoup4
```
然后,你可以使用以下代码来爬取天气数据:
```python
import requests
from bs4 import BeautifulSoup
# 发送HTTP请求获取网页内容
url = "https://www.example.com" # 替换为天气网站的URL
response = requests.get(url)
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(response.content, "html.parser")
# 根据HTML结构提取天气数据
# 这里只是一个示例,具体的提取方法会根据网页结构而有所不同
temperature = soup.find("span", class_="temperature").text
humidity = soup.find("span", class_="humidity").text
# 打印天气数据
print("Temperature:", temperature)
print("Humidity:", humidity)
```
请注意,这只是一个简单的示例。实际的网页结构和提取方法可能会有所不同。你需要根据具体的天气网站来调整代码中的选择器和提取逻辑。
希望这个示例对你有帮助!如果你有任何进一步的问题,请随时问我。
使用jupyter notebook爬取网页数据
好的,让我来回答你的问题。
首先,你需要安装Python和Jupyter Notebook。如果你还没有安装它们,你可以从官方网站下载并安装。
接下来,你需要安装一些Python库来帮助你爬取网页数据。常用的库包括BeautifulSoup, requests和pandas。你可以使用以下命令来安装它们:
```
!pip install beautifulsoup4
!pip install requests
!pip install pandas
```
现在你可以开始爬取网页数据了。下面是一个简单的示例代码,它使用requests库从网页中获取HTML代码,然后使用BeautifulSoup库解析HTML代码,并将结果保存为CSV文件。
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 发送请求获取HTML代码
url = 'https://www.example.com/'
response = requests.get(url)
html = response.content
# 解析HTML代码
soup = BeautifulSoup(html, 'html.parser')
title = soup.find('title').text
links = soup.find_all('a')
data = []
for link in links:
data.append((link.get('href'), link.text))
# 将结果保存为CSV文件
df = pd.DataFrame(data, columns=['url', 'text'])
df.to_csv('data.csv', index=False)
```
这只是一个简单的示例,你可以根据自己的需要进行修改和扩展。同时,你需要注意爬取网页数据的法律和道德问题,不要进行任何不合法或不道德的行为。
阅读全文
相关推荐
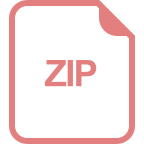
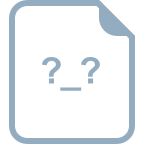
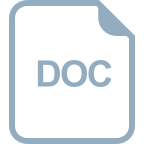












