补全createThread函数,使用pthread_create函数创建线程,并将start_routine作为线程处理函数,arg作为线程处理函数的参数,同时将创建成功的线程ID作为createThread函数的返回值。 测试说明
时间: 2024-11-22 07:42:06 浏览: 4
在C语言中,使用POSIX线程库(pthread)创建线程通常需要包含头文件`#include <pthread.h>`,然后编写如下的`createThread`函数:
```c
#include <pthread.h>
#include <stdio.h>
typedef void (*thread_routine)(void *arg); // 定义线程处理函数的类型
// 创建线程函数
pthread_t createThread(thread_routine start_routine, void *arg) {
pthread_t thread_id; // 存储线程ID
pthread_attr_t attr; // 线程属性
// 初始化线程属性
pthread_attr_init(&attr);
// 设置线程属性(例如堆栈大小、优先级等)
// 在这里假设没有特殊设置,仅创建线程
pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_DETACHED); // 创建非挂起的线程
// 创建线程
if (pthread_create(&thread_id, &attr, start_routine, arg)) {
printf("Error creating thread: %s\n", strerror(errno));
return PTHREAD_FAILURE; // 返回错误标识
}
// 清理线程属性,不是必需的,但如果要释放资源,可以这样做
pthread_attr_destroy(&attr);
return thread_id; // 返回成功创建的线程ID
}
// 测试示例线程处理函数
void start_routine(void *param) {
printf("Thread ID: %lu, Parameter: %p\n", (unsigned long)pthread_self(), param);
}
int main() {
pthread_t my_thread;
void *dummy_arg = NULL;
// 调用createThread函数创建线程
my_thread = createThread(start_routine, dummy_arg);
// 检查是否创建成功
if (my_thread != PTHREAD_FAILURE) {
printf("Thread created successfully with ID: %ld\n", (long)my_thread);
} else {
printf("Thread creation failed.\n");
}
return 0;
}
```
在这个例子中,`createThread`函数接受一个指向`thread_routine`类型的指针和一个用户提供的参数,然后调用`pthread_create`创建新线程。线程处理函数`start_routine`接收传入的参数并进行相应的操作。
阅读全文
相关推荐
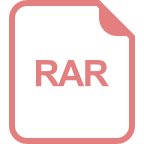
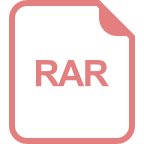
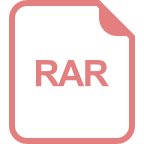











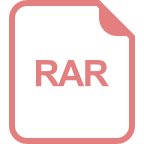
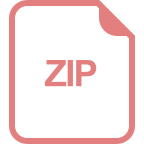
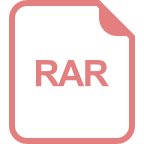
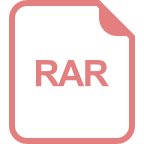
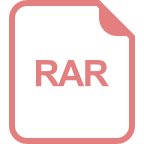