用c++写一个头尾交换的单链表
时间: 2024-10-22 15:12:27 浏览: 26
在C++中,我们可以使用指针和临时变量来实现链表的头部和尾部元素的交换。这里是一个简单的示例,假设我们有一个`ListNode`结构体代表链表节点:
```cpp
#include <iostream>
// 定义链表节点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 交换链表头部和尾部元素
ListNode* swapHeadAndTail(ListNode* head) {
if (head == nullptr || head->next == nullptr) { // 如果链表为空或只有一个元素,无需交换
return head;
}
ListNode* tail = head; // 初始化tail指向头节点
while (tail->next != nullptr) { // 找到尾部节点
tail = tail->next;
}
ListNode* temp = head->next; // 创建一个临时指针保存原尾部节点
head->next = tail; // 将原尾部接到头位置
tail->next = temp; // 尾部原来的头节点连接到新的尾部
return tail; // 返回新的尾部节点
}
// 测试函数
void printList(ListNode* head) {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->val << " -> ";
current = current->next;
}
std::cout << "nullptr" << std::endl;
}
int main() {
// 创建一个测试链表:1->2->3->4
ListNode* list = new ListNode(1);
list->next = new ListNode(2);
list->next->next = new ListNode(3);
list->next->next->next = new ListNode(4);
std::cout << "Original List: ";
printList(list);
list = swapHeadAndTail(list); // 调用函数交换头尾
std::cout << "After Swap: ";
printList(list);
return 0;
}
```
运行这个程序后,原始链表 `1->2->3->4` 会变成 `4->3->2->1`。
阅读全文
相关推荐
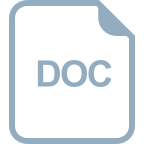
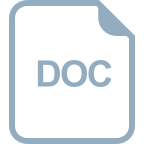
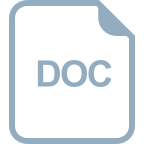
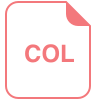
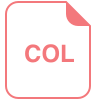
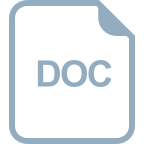
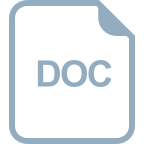
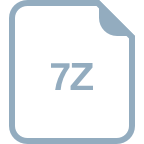
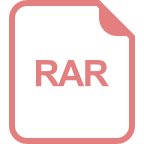
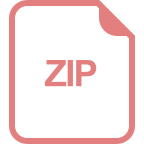
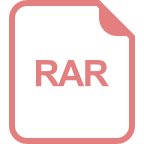
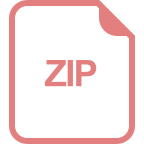
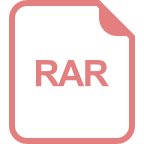
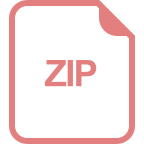
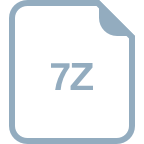
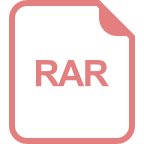
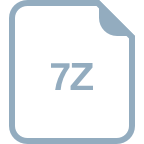
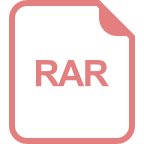
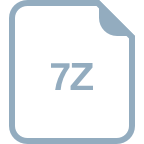
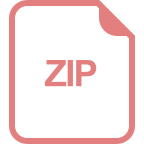