pip install segmentation_models_pytorch指定阿里云
时间: 2024-11-26 10:20:53 浏览: 56
`pip install segmentation_models_pytorch` 是用于安装 PyTorch 中的图像分割模型库 Segmentation Models 的命令。如果你想要从阿里云镜像安装这个包,你可以通过设置 `pip` 的源地址来指向阿里云的 Python 包仓库。
首先,你需要确保你的系统已经配置了阿里云的镜像源。在中国大陆地区,可以使用阿里云的 `mirrors.aliyun.com` 镜像。然后,你可以按照以下步骤操作:
1. 打开终端或命令提示符。
2. 如果还没有设置 `pip` 的源,可以添加阿里云的源:
```bash
pip config set global.index-url https://mirrors.aliyun.com/pypi/simple/
```
3. 接下来,更新 `pip` 到最新版本,以确保可以从新源获取软件包:
```bash
pip install --upgrade pip
```
4. 最后,你可以继续安装 `segmentation_models_pytorch`:
```bash
pip install segmentation_models_pytorch
```
记得替换 `https://mirrors.aliyun.com/pypi/simple/` 为你所在地区的阿里云镜像链接,如果需要。
相关问题
conda安装segmentation_models_pytorch
在Conda环境中安装`segmentation_models_pytorch`是一个常见的Python深度学习库管理步骤。首先,你需要确保已经安装了Anaconda环境管理和Miniconda。接下来,你可以按照以下步骤操作:
1. **激活虚拟环境**(如果你还没有创建,需要先创建一个):
```
conda create -n your_env_name python=3.x # 根据需求选择Python版本
conda activate your_env_name
```
2. **安装pip**(因为Conda有自己的包管理,但在某些情况下pip可能更方便):
```
conda install pip
```
3. **通过pip安装`segmentation_models_pytorch`**:
```
pip install segmentation-models-pytorch
```
或者如果想要从GitHub克隆并安装源码:
```
git clone https://github.com/qubvel/segmentation_models.pytorch.git
cd segmentation_models.pytorch
pip install .
```
4. 确认安装完成:
```
python -c "import segmentation_models; print(segmentation_models.__version__)"
```
使用segmentation_models_pytorch实现单模型训练
### 回答1:
segmentation_models_pytorch 是一个基于 PyTorch 的图像分割库,可以用来训练语义分割模型。下面是使用 segmentation_models_pytorch 实现单模型训练的基本步骤:
1. 安装 segmentation_models_pytorch 和其依赖项:
```
pip install segmentation-models-pytorch
```
2. 加载数据集并进行预处理。可以使用 torchvision 或者其他图像处理库加载数据集,并对数据进行预处理,如裁剪、缩放、归一化等操作。
3. 定义模型。使用 segmentation_models_pytorch 中提供的模型类(如 UNet、FPN、PSPNet 等)来定义模型。
```python
import segmentation_models_pytorch as smp
model = smp.Unet(
encoder_name="resnet34", # 使用 ResNet34 作为编码器
encoder_weights="imagenet", # 加载预训练权重
in_channels=3, # 输入通道数
classes=2, # 分类数
)
```
4. 定义损失函数和优化器。可以选择使用交叉熵损失函数和 Adam 优化器。
```python
import torch.nn as nn
import torch.optim as optim
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
5. 训练模型。使用 DataLoader 加载数据集,并对模型进行训练。
```python
from torch.utils.data import DataLoader
train_loader = DataLoader(dataset, batch_size=4, shuffle=True)
for epoch in range(num_epochs):
running_loss = 0.0
for i, data in enumerate(train_loader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}, Loss: {running_loss/len(train_loader)}")
```
6. 保存模型。训练完毕后,可以使用 torch.save() 方法将模型保存到本地。
```python
torch.save(model.state_dict(), "model.pth")
```
### 回答2:
segmentation_models_pytorch是一个基于PyTorch实现的语义分割模型库。使用segmentation_models_pytorch实现单模型训练可以通过以下步骤完成。
首先,安装segmentation_models_pytorch库。可以通过pip install segmentation_models_pytorch命令来安装。
导入所需的库和模型。常用的库包括torch,torchvision和segmentation_models_pytorch。可以使用以下命令导入库:
```python
import torch
import torchvision.transforms as transforms
import segmentation_models_pytorch as smp
```
加载和预处理训练数据。可以使用torchvision中的transforms来定义一系列的数据预处理操作,例如裁剪、缩放和标准化等。之后,使用torch.utils.data.DataLoader来加载和批量处理数据。
定义模型架构。可以选择使用segmentation_models_pytorch中预定义的模型架构,例如UNet、PSPNet和DeepLab等。根据任务需求选择合适的模型,并初始化相关参数。
定义优化器和损失函数。常见的优化器有Adam和SGD等,损失函数常选择交叉熵损失函数。可以使用torch.optim中的函数来定义优化器,使用torch.nn中的损失函数来定义损失函数。
进行模型训练。使用torch.utils.data.DataLoader加载训练数据集,并迭代训练数据集中的每个批次。将批次数据输入模型中进行前向传播,获取模型的输出。计算损失,并进行反向传播更新模型的参数。重复以上步骤直到达到预定的训练轮数或达到设定的训练目标。
保存和加载训练好的模型。可以使用torch.save函数将训练好的模型保存到指定的文件路径,使用torch.load函数加载保存的模型文件。
以上是使用segmentation_models_pytorch实现单模型训练的基本步骤。根据具体任务和数据的不同,可能还需要进行一些细节操作,例如数据增强、学习率调整和模型评估等。
### 回答3:
segmentation_models_pytorch是一个基于PyTorch的分割模型训练库,可以应用于图像分割任务。下面我将介绍如何使用segmentation_models_pytorch实现单模型训练。
首先,我们需要安装segmentation_models_pytorch库。可以使用pip命令进行安装:
```
pip install segmentation-models-pytorch
```
在训练之前,我们需要准备好训练数据和标签。通常情况下,训练数据是一些图像,标签则是对应每个像素点的分类或分割结果。
接下来,我们需要导入所需的库:
```
import segmentation_models_pytorch as smp
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader, Dataset
```
然后,我们需要创建一个自定义的数据集类,该类继承自torch.utils.data.Dataset类,并实现__len__和__getitem__方法,用于加载和处理数据。
接着,我们可以选择一个合适的分割模型,比如Unet、FPN等。这些模型可以通过调用smp库中的函数进行初始化,比如:
```
model = smp.Unet(
encoder_name="resnet34",
encoder_weights="imagenet",
classes=1,
activation='sigmoid'
)
```
在这里,我们选择了一个使用ResNet-34作为编码器、预训练权重为ImageNet数据集、分类数为1(二分类问题)的Unet模型。
然后,我们可以定义损失函数和优化器:
```
criterion = nn.BCELoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
接着,我们可以进行训练循环,依次迭代数据进行训练和优化:
```
for epoch in range(num_epochs):
for batch in dataloader:
inputs, labels = batch
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
最后,我们可以保存模型并在需要预测时加载模型进行测试:
```
torch.save(model.state_dict(), "segmentation_model.pt")
model.load_state_dict(torch.load("segmentation_model.pt"))
```
以上就是使用segmentation_models_pytorch实现单模型训练的过程。根据具体任务需求,你也可以调整模型、损失函数、优化器等参数来进行更灵活的训练。
阅读全文
相关推荐
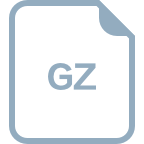















