数据结构中试编写一个算法,可以算出循环链表的长度,用c语言编写
时间: 2024-09-23 21:15:25 浏览: 39
在C语言中,计算循环链表的长度需要遍历整个链表,但由于链表节点可能会形成环路,常规的递归或单次遍历无法完成这个任务。通常我们会使用“快慢指针”或者“两个指针”的技巧来解决这个问题。下面是一个简单的示例:
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
// 判断链表是否为循环链表
int hasCycle(Node* head) {
if (head == NULL || head->next == NULL) return 0;
Node *slow = head; // 慢指针每次移动一步
Node *fast = head->next; // 快指针每次移动两步
while (slow != fast) {
if (fast == NULL || fast->next == NULL) return 0; // 如果快指针到达了NULL,则不是循环链表
slow = slow->next;
fast = fast->next->next;
if (slow == fast) break; // 如果快慢相遇,说明形成了环
}
return 1; // 如果到此位置,说明是循环链表
}
// 计算循环链表的实际长度
int getLoopLength(Node *head) {
if (!hasCycle(head)) return 0; // 非循环链表直接返回0
Node *lengthNode = head;
int length = 0;
while (1) {
length++;
lengthNode = lengthNode->next;
if (lengthNode == head) break; // 找到了循环起点,退出循环
}
return length; // 返回实际的链表长度
}
// 测试函数
void test() {
// 创建一个循环链表...
Node *node1 = (Node*)malloc(sizeof(Node));
Node *node2 = (Node*)malloc(sizeof(Node));
Node *node3 = (Node*)malloc(sizeof(Node));
node1->data = 1;
node1->next = node2;
node2->data = 2;
node2->next = node3;
node3->data = 3;
node3->next = node2; // 设置循环
printf("循环链表长度: %d\n", getLoopLength(node1));
}
int main() {
test();
return 0;
}
```
相关推荐
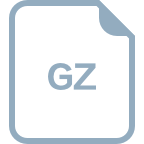
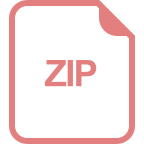
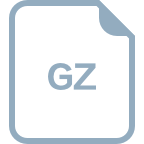














