C语言拷贝数组指定索引数据到新数组
时间: 2024-10-06 13:01:32 浏览: 17
在C语言中,如果你想从一个数组复制特定索引范围的数据到另一个新的数组,你可以通过遍历并选择源数组中指定索引位置的数据来完成。这里是一个简单的示例:
```c
#include <stdio.h>
// 函数声明
void copyArray(int source[], int destination[], int start_index, int length);
int main() {
int source[5] = {1, 2, 3, 4, 5}; // 源数组
int destination[5]; // 目标数组
int start_index = 1; // 起始索引
int length = 3; // 需要复制的元素个数
// 调用函数,将source数组中start_index开始的length个元素复制到destination数组
copyArray(source, destination, start_index, length);
// 打印目标数组内容
for (int i = 0; i < length; i++) {
printf("Destination array at index %d: %d\n", i, destination[i]);
}
return 0;
}
// 定义copyArray函数
void copyArray(int source[], int destination[], int start_index, int length) {
if (start_index + length <= sizeof(source)) { // 确保不会越界
for (int i = 0; i < length; i++) {
destination[start_index + i] = source[start_index + i]; // 复制元素
}
} else {
printf("Error: Index out of bounds.\n");
}
}
```
在这个例子中,`copyArray`函数接受两个数组(源和目的地),起始索引和需要复制的元素个数。它只复制指定范围内(不包括边界)的数据,并检查是否越界。
阅读全文
相关推荐
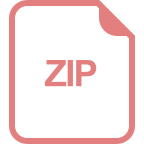
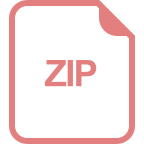
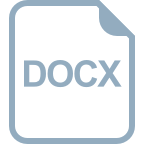















