图片浏览器python
时间: 2023-10-29 07:57:59 浏览: 44
为了实现一个图片浏览器,可以使用Python的Tkinter库来创建用户界面,并使用glob库来获取文件夹中的所有图片文件。可以使用PIL库来处理图片,并使用ImageTk库将图片转换为Tkinter可用的格式。
下面是一个简单的示例代码,可以帮助你开始编写一个图片浏览器:
```python
import tkinter as tk
from PIL import Image, ImageTk
import glob
class ImageBrowser(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.images = []
self.current_index = 0
self.create_widgets()
self.load_images()
def create_widgets(self):
self.image_label = tk.Label(self)
self.image_label.pack()
self.prev_button = tk.Button(self, text="Previous", command=self.show_previous_image)
self.prev_button.pack(side="left")
self.next_button = tk.Button(self, text="Next", command=self.show_next_image)
self.next_button.pack(side="right")
def load_images(self):
image_files = glob.glob("path/to/image/folder/*.jpg") # 替换为你的图片文件夹路径和文件类型
for file in image_files:
image = Image.open(file)
self.images.append(image)
self.show_image()
def show_image(self):
image = self.images[self.current_index]
image = image.resize((400, 400)) # 可以调整图片大小
photo = ImageTk.PhotoImage(image)
self.image_label.configure(image=photo)
self.image_label.image = photo
def show_previous_image(self):
self.current_index = (self.current_index - 1) % len(self.images)
self.show_image()
def show_next_image(self):
self.current_index = (self.current_index + 1) % len(self.images)
self.show_image()
root = tk.Tk()
app = ImageBrowser(master=root)
app.mainloop()
```
这段代码创建了一个名为`ImageBrowser`的类,继承自`tkinter`的`Frame`类。在`__init__`方法中,初始化了界面和变量,并调用`load_images`方法加载图片。
`create_widgets`方法创建了界面上的控件,包括一个`Label`用于显示图片,和两个`Button`用于切换上一张和下一张图片。
`load_images`方法使用`glob`库获取指定文件夹中的所有图片文件,并使用`PIL`库打开并保存在`self.images`列表中。
`show_image`方法根据当前索引显示对应的图片,并使用`configure`方法将图片设置为`Label`的内容。
`show_previous_image`和`show_next_image`方法分别用于显示上一张和下一张图片,并通过更新索引调用`show_image`方法来刷新界面。
最后,创建一个`Tk`实例并实例化`ImageBrowser`类,通过调用`mainloop`方法进入主循环,使程序开始运行。
你可以根据需要修改代码中的路径和其他细节来适应你的需求。希望这个例子对你有所帮助!
相关推荐
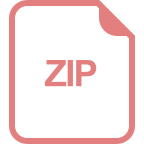
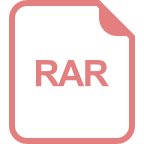
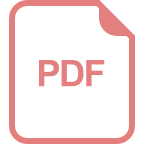
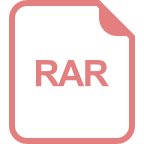
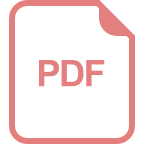
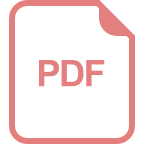
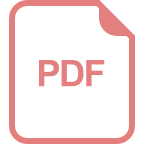
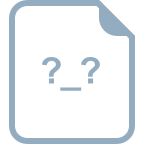
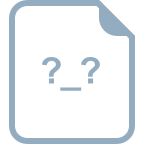