用C语言设计一个线性表,采用顺序存储结构,插入一个元素
时间: 2024-09-27 07:16:04 浏览: 54
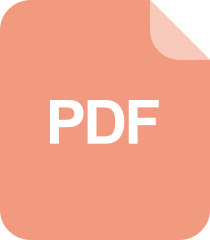
C语言线性表顺序存储结构实例详解

在C语言中,设计一个简单的顺序存储结构(如数组)的线性表,可以按照以下步骤插入一个新元素:
首先,你需要定义一个数据结构来表示线性表的节点,以及一个用于保存表长度的变量:
```c
#include <stdio.h>
// 定义节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 定义线性表结构体
typedef struct List {
Node* head; // 表头指针
int size; // 当前元素个数
} List;
```
接下来,创建一个函数来插入新元素:
```c
void insertElement(List* list, int value) {
// 如果列表为空,将新元素作为第一个元素添加
if (list->head == NULL) {
list->head = (Node*)malloc(sizeof(Node));
list->head->data = value;
list->head->next = NULL;
list->size = 1;
} else {
// 找到最后一个元素并插入
Node* current = list->head;
while (current->next != NULL) {
current = current->next;
}
Node* newNode = (Node*)malloc(sizeof(Node)); // 新节点分配内存
newNode->data = value;
newNode->next = NULL;
current->next = newNode; // 插入新节点
list->size++; // 更新元素个数
}
}
```
最后,在主程序中使用这个函数:
```c
int main() {
List myList;
myList.head = NULL;
myList.size = 0;
// 测试插入操作
insertElement(&myList, 5);
insertElement(&myList, 10);
insertElement(&myList, 15);
return 0;
}
```
阅读全文
相关推荐
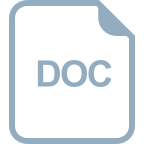
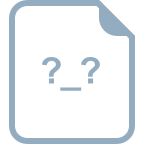







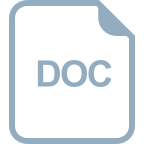
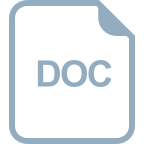
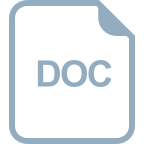
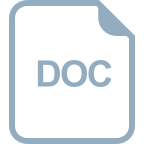



