写出解决问题的程序,使用Python
时间: 2024-10-29 19:03:49 浏览: 5
为了实现启发式搜索算法解决八数码难题,我们可以按照以下步骤编写Python代码:
### 八数码问题描述
- **目标状态**:`[1, 2, 3, 8, 0, 4, 7, 6, 5]`
- **初始状态**:可以通过用户输入来设置
### 数据结构
- 使用二维列表表示棋盘状态
- `OPEN` 表和 `CLOSED` 表可以分别用优先队列(最小堆)和列表来实现
### 估价函数
- 总代价函数 \( f(n) = g(n) + h(n) \),其中 \( g(n) \) 是从初始状态到当前状态的实际路径代价,\( h(n) \) 是启发式估计代价
### Python 实现
```python
import heapq
# 定义目标状态
GOAL_STATE = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
# 计算曼哈顿距离
def manhattan_distance(state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
target_i, target_j = divmod(state[i][j] - 1, 3)
distance += abs(i - target_i) + abs(j - target_j)
return distance
# 获取空格位置
def get_blank_position(state):
for i in range(3):
for j in range(3):
if state[i][j] == 0:
return i, j
# 生成所有可能的后续状态
def generate_successors(state):
successors = []
blank_i, blank_j = get_blank_position(state)
directions = [(0, 1), (1, 0), (-1, 0), (0, -1)]
for di, dj in directions:
new_i, new_j = blank_i + di, blank_j + dj
if 0 <= new_i < 3 and 0 <= new_j < 3:
new_state = [row[:] for row in state]
new_state[blank_i][blank_j], new_state[new_i][new_j] = new_state[new_i][new_j], new_state[blank_i][blank_j]
successors.append(new_state)
return successors
# A* 搜索算法
def a_star_search(initial_state):
# 初始化 OPEN 表和 CLOSED 表
open_list = []
closed_set = set()
# 将初始状态加入 OPEN 表
initial_f = manhattan_distance(initial_state)
heapq.heappush(open_list, (initial_f, 0, initial_state, []))
while open_list:
_, g, current_state, path = heapq.heappop(open_list)
if current_state == GOAL_STATE:
return path + [current_state]
if tuple(map(tuple, current_state)) in closed_set:
continue
closed_set.add(tuple(map(tuple, current_state)))
for successor in generate_successors(current_state):
if tuple(map(tuple, successor)) not in closed_set:
new_g = g + 1
new_f = new_g + manhattan_distance(successor)
heapq.heappush(open_list, (new_f, new_g, successor, path + [current_state]))
return None
# 主函数
if __name__ == "__main__":
# 输入初始状态
initial_state = []
print("请输入初始状态,每行三个数字,空格用0表示:")
for _ in range(3):
row = list(map(int, input().split()))
initial_state.append(row)
# 搜索并输出结果
solution = a_star_search(initial_state)
if solution:
print("找到解决方案:")
for step in solution:
for row in step:
print(row)
print()
else:
print("没有找到解决方案。")
```
### 说明
1. **曼哈顿距离**:用于计算每个数字离目标位置的距离。
2. **A* 搜索算法**:结合了实际代价 \( g(n) \) 和启发式代价 \( h(n) \) 来选择最优路径。
3. **优先队列**:使用 `heapq` 模块实现,保证每次取出的是当前最优的节点。
4. **用户输入**:通过控制台输入初始状态,方便测试不同的起始点。
希望这段代码能帮助你理解和实现启发式搜索算法解决八数码难题。如果有任何问题,请随时提问!
阅读全文
相关推荐
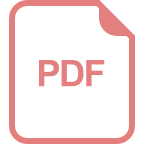
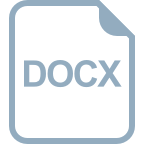

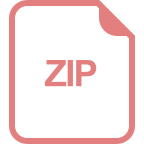
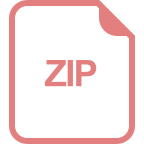
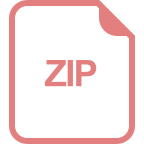
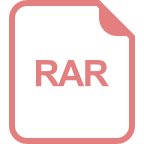
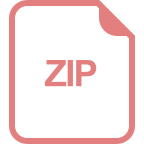
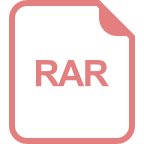
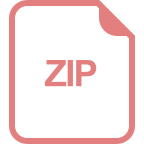
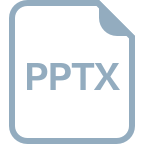
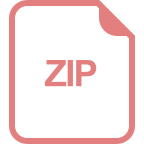
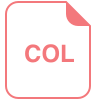




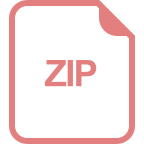