c++字符串分割字符串
时间: 2023-11-27 12:51:07 浏览: 86
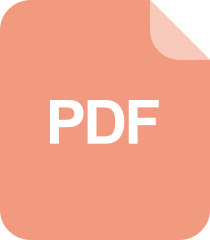
C++常用字符串分割方法实例汇总

在C++中,可以使用多种方法来分割字符串。以下是其中几种常见的方法:
1. 使用stringstream:
```cpp
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
int main() {
std::string str = "hello,world,this,is,a,test";
std::vector<std::string> tokens;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, ',')) {
tokens.push_back(token);
}
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
2. 使用C++标准库的字符串处理函数:
```cpp
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "hello,world,this,is,a,test";
std::vector<std::string> tokens;
size_t pos = 0;
std::string token;
while ((pos = str.find(',')) != std::string::npos) {
token = str.substr(0, pos);
tokens.push_back(token);
str.erase(0, pos + 1);
}
tokens.push_back(str); // 添加最后一个分割后的子串
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
3. 使用Boost库中的字符串分割函数:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <boost/algorithm/string.hpp>
int main() {
std::string str = "hello,world,this,is,a,test";
std::vector<std::string> tokens;
boost::split(tokens, str, boost::is_any_of(","));
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
以上是几种常见的字符串分割方法,你可以根据自己的需求和喜好选择其中的一种。
阅读全文
相关推荐
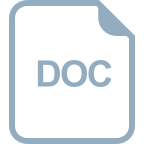
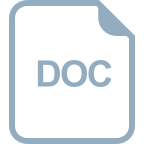
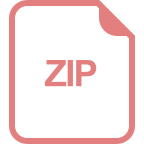




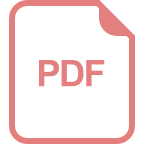
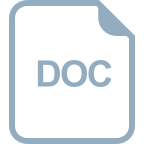
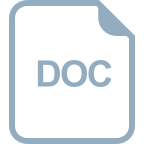




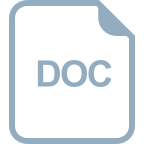
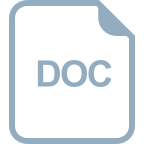
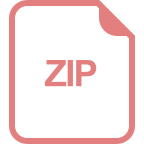