用C++利用线程池写一个从vstor传输文件到亚马逊云的代码
时间: 2024-03-06 12:48:06 浏览: 123
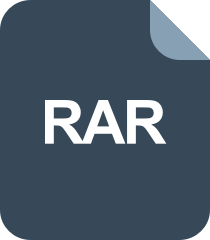
c++用线程文件传输

以下是一个简单的C++代码示例,演示了如何使用线程池来传输文件从vstor到亚马逊云。请注意,这只是一个简单的示例,您需要根据您的具体情况进行适当的更改和调整。
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <future>
#include <queue>
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <aws/s3/model/Object.h>
using namespace std;
using namespace Aws::S3;
using namespace Aws::S3::Model;
// 定义线程池类
class ThreadPool {
public:
ThreadPool(size_t num_threads) {
for (size_t i = 0; i < num_threads; ++i) {
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock, [this] {
return !this->stop || !this->tasks.empty();
});
if (this->stop && this->tasks.empty()) {
return;
}
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread &worker : workers) {
worker.join();
}
}
template<class F, class... Args>
auto enqueue(F &&f, Args &&... args) -> std::future<typename std::result_of<F(Args...)>::type> {
using return_type = typename std::result_of<F(Args...)>::type;
auto task = std::make_shared<std::packaged_task<return_type()>>(std::bind(std::forward<F>(f), std::forward<Args>(args)...));
std::future<return_type> res = task->get_future();
{
std::unique_lock<std::mutex> lock(queue_mutex);
if (stop) {
throw std::runtime_error("enqueue on stopped ThreadPool");
}
tasks.emplace([task]() { (*task)(); });
}
condition.notify_one();
return res;
}
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop = false;
};
// 从vstor读取文件
vector<char> read_from_vstor(const string &file_path) {
vector<char> buffer;
ifstream file(file_path, ios::binary);
if (file) {
file.seekg(0, ios::end);
buffer.resize(file.tellg());
file.seekg(0, ios::beg);
file.read(&buffer[0], buffer.size());
}
return buffer;
}
// 将文件上传到亚马逊云
void upload_to_s3(const string &file_path, const string &bucket_name, const string &key_name) {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
S3Client s3_client;
auto request = PutObjectRequest();
request.SetBucket(bucket_name.c_str());
request.SetKey(key_name.c_str());
auto input_data = read_from_vstor(file_path);
auto input_data_stream = Aws::MakeShared<Aws::StringStream>("SampleAllocationTag",
std::string(reinterpret_cast<const char *>(input_data.data()), input_data.size()));
request.SetBody(input_data_stream);
auto put_object_outcome = s3_client.PutObject(request);
if (!put_object_outcome.IsSuccess()) {
auto error = put_object_outcome.GetError();
std::cout << "ERROR: " << error.GetMessage() << std::endl;
}
}
Aws::ShutdownAPI(options);
}
int main() {
const string file_path = "your_file_path";
const string bucket_name = "your_bucket_name";
const string key_name = "your_key_name";
const size_t num_threads = 4;
ThreadPool pool(num_threads);
// 将任务放入线程池中
auto upload_task = [&file_path, &bucket_name, &key_name] { upload_to_s3(file_path, bucket_name, key_name); };
auto result = pool.enqueue(upload_task);
// 等待任务完成
result.get();
return 0;
}
```
在这个示例中,我们使用了AWS SDK来上传文件到亚马逊S3。您需要根据您的具体情况进行相应的更改和调整,例如替换文件路径,桶名称和密钥名称。
阅读全文
相关推荐

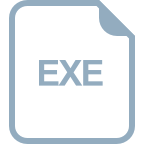
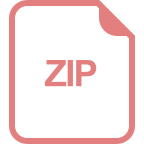
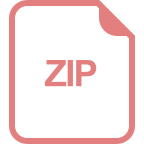
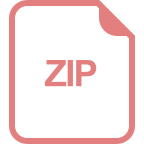
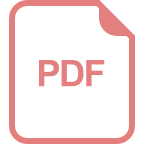
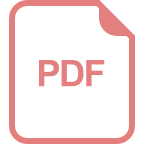
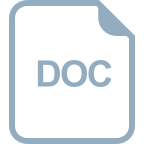
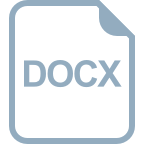
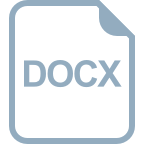
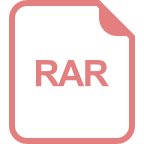
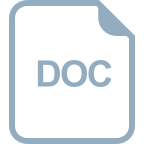



